485轮询主从通讯c语言代码
时间: 2023-09-17 07:06:01 浏览: 121
### 回答1:
轮询主从通讯是指在主从通信中,主设备通过不断地轮询从设备的状态,以实现数据传输的一种通信方式。下面是一个基本的C语言示例代码,用于实现轮询主从通信:
主设备代码:
```c
#include <stdio.h>
#include <stdbool.h>
#define SLAVE_READY 0x01 // 从设备准备好的状态码
int main() {
bool slave_ready = false;
while (!slave_ready) {
// 轮询从设备状态
// 假设通过I2C总线通信
int slave_status = i2c_read_status();
if (slave_status == SLAVE_READY) {
slave_ready = true;
printf("Slave device is ready.\n");
}
}
// 向从设备发送数据
// 假设通过I2C总线通信
i2c_send_data();
return 0;
}
```
从设备代码:
```c
#include <stdio.h>
#define SLAVE_READY 0x01 // 从设备准备好的状态码
int main() {
// 从设备准备好后发送状态码
// 假设通过I2C总线通信
i2c_send_status(SLAVE_READY);
// 接收主设备发送的数据
// 假设通过I2C总线通信
i2c_receive_data();
return 0;
}
```
需要注意的是,上述示例代码只是一个简单的示例,实际情况可能会更加复杂。具体实现方式还需根据具体的通信协议和硬件设备进行调整。
### 回答2:
485轮询主从通讯c语言代码的实现可以借助于串行通信接口(如UART)进行数据传输。以下是一个简单的示例代码:
主设备代码示例:
``` c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd; // 串行通信接口文件描述符
struct termios options; // 储存串行通信接口配置参数
// 打开串行通信接口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串行通信接口");
exit(1);
}
// 配置串行通信接口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 等待485从设备准备好
usleep(10000);
// 向485从设备发送数据(假设发送的是字符A)
write(fd, "A", 1);
// 读取从设备返回的数据
char buffer[10];
read(fd, buffer, sizeof(buffer));
// 打印接收到的数据
printf("接收到的数据: %s\n", buffer);
// 关闭串行通信接口
close(fd);
return 0;
}
```
从设备代码示例:
``` c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main() {
int fd; // 串行通信接口文件描述符
struct termios options; // 储存串行通信接口配置参数
// 打开串行通信接口
fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (fd == -1) {
perror("无法打开串行通信接口");
exit(1);
}
// 配置串行通信接口
tcgetattr(fd, &options);
cfsetispeed(&options, B9600);
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
tcsetattr(fd, TCSANOW, &options);
// 接收主设备发送的数据
char buffer[10];
read(fd, buffer, sizeof(buffer));
// 向主设备发送返回数据(假设发送的是字符B)
write(fd, "B", 1);
// 关闭串行通信接口
close(fd);
return 0;
}
```
以上是一个简单的485轮询主从通信的C语言代码示例。实际使用时,需根据具体的硬件设备和通信环境进行相应的配置和适配。
### 回答3:
以下是一个用C语言编写的485轮询主从通讯的示例代码:
主机端代码:
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define BAUDRATE B9600 // 485通讯波特率
#define DEVICE "/dev/ttyUSB0" // 485通讯设备
#define BUF_SIZE 512 // 数据缓冲区大小
int main() {
int fd, res;
struct termios oldtio, newtio;
char buf[BUF_SIZE];
// 打开串口设备
fd = open(DEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("无法打开串口设备");
exit(1);
}
// 保存当前终端配置
tcgetattr(fd, &oldtio);
// 清空新的终端配置
memset(&newtio, 0, sizeof(newtio));
// 设置终端控制模式,485通讯一般为非规范模式
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = 0;
newtio.c_oflag = 0;
newtio.c_lflag = 0;
newtio.c_cc[VTIME] = 0;
newtio.c_cc[VMIN] = 1;
// 应用新的终端配置
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &newtio);
// 轮询发送与接收数据
while (1) {
// 向从机发送数据
res = write(fd, "Hello", 5);
if (res != 5) {
perror("发送数据失败");
exit(1);
}
sleep(1); // 间隔1秒钟
// 接收从机返回的数据
res = read(fd, buf, BUF_SIZE);
if (res > 0) {
printf("收到从机返回的数据:%s\n", buf);
}
}
// 恢复终端配置
tcsetattr(fd, TCSANOW, &oldtio);
// 关闭串口设备
close(fd);
return 0;
}
从机端代码:
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define BAUDRATE B9600 // 485通讯波特率
#define DEVICE "/dev/ttyUSB0" // 485通讯设备
#define BUF_SIZE 512 // 数据缓冲区大小
int main() {
int fd, res;
struct termios oldtio, newtio;
char buf[BUF_SIZE];
// 打开串口设备
fd = open(DEVICE, O_RDWR | O_NOCTTY);
if (fd < 0) {
perror("无法打开串口设备");
exit(1);
}
// 保存当前终端配置
tcgetattr(fd, &oldtio);
// 清空新的终端配置
memset(&newtio, 0, sizeof(newtio));
// 设置终端控制模式,485通讯一般为非规范模式
newtio.c_cflag = BAUDRATE | CS8 | CLOCAL | CREAD;
newtio.c_iflag = 0;
newtio.c_oflag = 0;
newtio.c_lflag = 0;
newtio.c_cc[VTIME] = 0;
newtio.c_cc[VMIN] = 1;
// 应用新的终端配置
tcflush(fd, TCIFLUSH);
tcsetattr(fd, TCSANOW, &newtio);
// 轮询接收与发送数据
while (1) {
// 接收主机发送的数据
res = read(fd, buf, BUF_SIZE);
if (res > 0) {
printf("收到主机发送的数据:%s\n", buf);
}
// 向主机发送数据
res = write(fd, "World", 5);
if (res != 5) {
perror("发送数据失败");
exit(1);
}
sleep(1); // 间隔1秒钟
}
// 恢复终端配置
tcsetattr(fd, TCSANOW, &oldtio);
// 关闭串口设备
close(fd);
return 0;
}
以上代码实现了一个简单的485轮询通讯示例,主机端和从机端分别进行发送数据和接收数据的操作,并通过串口进行通信。在示例中,主机端每隔1秒向从机端发送"Hello",从机端接收到数据后打印,然后向主机端发送"World",主机端接收到数据后打印。这样主机端和从机端就能够互相通信了。
相关推荐
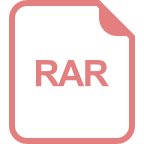
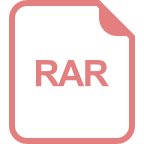
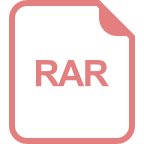


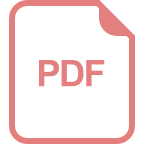
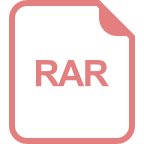
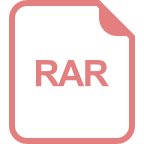
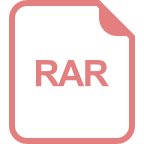
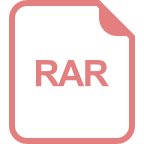
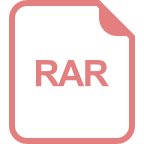
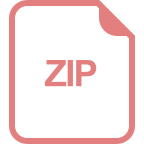
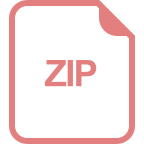
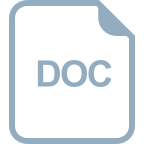
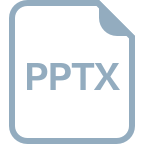
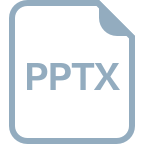
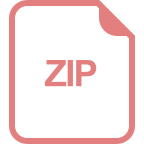