Android SimpleAdapter 实现购物车实例
时间: 2023-07-31 18:04:44 浏览: 51
好的,下面是一个简单的Android购物车示例,使用SimpleAdapter实现:
1. 首先,在布局文件中添加一个ListView控件,如下所示:
```
<ListView
android:id="@+id/list_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
2. 在Activity中,定义商品数据和购物车数据:
```
private List<Map<String, Object>> goodsList = new ArrayList<>();
private List<Map<String, Object>> cartList = new ArrayList<>();
private double totalPrice = 0;
```
3. 在onCreate方法中初始化商品数据和SimpleAdapter:
```
SimpleAdapter adapter = new SimpleAdapter(this, goodsList,
R.layout.item_goods, new String[] {"name", "price", "add"},
new int[] {R.id.tv_name, R.id.tv_price, R.id.btn_add});
adapter.setViewBinder(new SimpleAdapter.ViewBinder() {
@Override
public boolean setViewValue(View view, Object data, String textRepresentation) {
if (view instanceof Button) {
final Map<String, Object> item = (Map<String, Object>) view.getTag();
((Button) view).setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
addToCart(item);
}
});
return true;
}
return false;
}
});
// 初始化商品数据
Map<String, Object> item1 = new HashMap<>();
item1.put("name", "商品1");
item1.put("price", 10.0);
item1.put("add", "加入购物车");
goodsList.add(item1);
Map<String, Object> item2 = new HashMap<>();
item2.put("name", "商品2");
item2.put("price", 20.0);
item2.put("add", "加入购物车");
goodsList.add(item2);
// 绑定数据
ListView listView = findViewById(R.id.list_view);
listView.setAdapter(adapter);
```
4. 定义addToCart方法,将商品添加到购物车中:
```
private void addToCart(Map<String, Object> item) {
// 判断购物车中是否已经存在该商品
boolean exist = false;
for (Map<String, Object> cartItem : cartList) {
if (cartItem.get("name").equals(item.get("name"))) {
int count = (int) cartItem.get("count");
cartItem.put("count", count + 1);
exist = true;
break;
}
}
// 如果购物车中不存在该商品,则添加新的购物车项
if (!exist) {
Map<String, Object> cartItem = new HashMap<>();
cartItem.put("name", item.get("name"));
cartItem.put("price", item.get("price"));
cartItem.put("count", 1);
cartList.add(cartItem);
}
// 更新购物车总价
totalPrice += (double) item.get("price");
}
```
5. 在Activity中定义一个显示购物车信息的方法:
```
private void showCartInfo() {
StringBuilder builder = new StringBuilder();
builder.append("总价:").append(totalPrice).append("\n");
for (Map<String, Object> cartItem : cartList) {
builder.append(cartItem.get("name")).append(" x ")
.append(cartItem.get("count")).append("\n");
}
AlertDialog.Builder dialogBuilder = new AlertDialog.Builder(this);
dialogBuilder.setMessage(builder.toString())
.setPositiveButton("去结算", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// TODO: 发送购物车数据到后台进行结算
}
})
.setNegativeButton("取消", null)
.show();
}
```
6. 在Activity中添加一个按钮,点击该按钮可以显示购物车信息:
```
Button btnCart = findViewById(R.id.btn_cart);
btnCart.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
showCartInfo();
}
});
```
7. 最后,创建一个item_goods.xml布局文件,用于显示商品项:
```
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:padding="16dp">
<TextView
android:id="@+id/tv_name"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:textSize="16sp"
android:textStyle="bold" />
<TextView
android:id="@+id/tv_price"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="16sp" />
<Button
android:id="@+id/btn_add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:tag=""
android:textSize="16sp" />
</LinearLayout>
```
这样,就实现了一个简单的Android购物车,使用SimpleAdapter实现商品列表和购物车列表的展示。
相关推荐
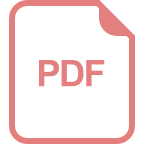














