#include <iostream> using namespace std; class Date { public: int year,month,day; Date(int year=2000,int month=1,int day=1):year(year),month(month),day(day) {} void print() { cout<<year<<","<<month<<","<<day; } }; class person { public: string Name; string Num; Date brith; person(string Name="张三", string Num="123456789123456789", int year=2000,int month=1,int day=1): Name(Name),Num(Num),brith(year,month,day) {} string GetName() { return Name; } string GetNum() { return Num; } Date Getbrith() { return brith; } }; class student: private person { private: int StNum; }; class teacher:public person { private: Date Time; }; class CTA :public student,public teacher { private: int No; }; int main() { person p1("张三","123456789123456789",2000,1,1); cout<<"序号"<<endl; cout<<" 1 "<<endl; cout<<" "<<p1.GetName()<<endl; cout<<" "<<p1.GetNum()<<endl; cout<<" "<<p1.Getbrith()<<endl; person p2("李四","123456789123456789",2001,1,1); cout<<" 2 "<<endl; cout<<" "<<p2.GetName()<<endl; cout<<" "<<p2.GetNum()<<endl; cout<<" "<<p2.Getbrith()<<endl; return 0; }no match for 'operator' and 'Date') cout怎么解决
时间: 2024-01-25 10:03:00 浏览: 140
在输出person类中的Date类型成员变量时,需要重载输出运算符<<,否则会出现no match for 'operator' and 'Date'的错误。可以在Date类中添加如下重载函数:
```
friend ostream& operator<<(ostream& os, const Date& date)
{
os << date.year << "," << date.month << "," << date.day;
return os;
}
```
然后在输出person类中的Date类型成员变量时,可以使用cout<<来调用该重载函数:
```
cout << " " << p1.Getbrith() << endl;
```
同样的,也需要在输出teacher类中的Date类型成员变量时进行相应的操作。
相关问题
分析下面的程序,写出其运行时的输出结果。#include<iostream> using namespace std; class Date
由于提供的代码片段不是一个完整的程序,而且缺少日期相关的类和成员函数,所以我无法直接分析并预测运行时的输出。但是,如果这个`Date`类是用来表示日期并且包含一些操作如打印年月日的方法,通常这样的代码结构可能会像这样:
```cpp
class Date {
public:
int year;
int month;
int day;
// 构造函数、getter和setter等
Date(int y, int m, int d) : year(y), month(m), day(d) {}
void printDate() { // 假设有一个打印日期的函数
cout << "Date: " << year << "-" << month << "-" << day << endl;
}
};
int main() {
Date today(2023, 1, 15);
today.printDate();
return 0;
}
```
在这种情况下,运行时的输出将是:
```
Date: 2023-1-15
```
如果你想了解更详细的结果,你需要提供`Date`类的所有内容以及它在`main`函数中的完整使用情况。
请设计日期 DATE 类。它包含三个私有数据成员:year (年)、month (月) 和 day (天),均为整数。 #include <iostream> using namespace std
为了设计DATE类,我们可以创建一个名为DATE的结构体或者类,包含year、month和day三个私有成员变量。这里以类的形式展示,因为类更便于封装和操作:
```cpp
class DATE {
private:
int year;
int month;
int day;
public:
// 构造函数,用于初始化日期
DATE(int y = 0, int m = 0, int d = 0) : year(y), month(m), day(d) {}
// 获取和设置年份
int getYear() const { return year; }
void setYear(int new_year) { year = new_year; }
// 获取和设置月份
int getMonth() const { return month; }
void setMonth(int new_month) { month = new_month; }
// 获取和设置日期
int getDay() const { return day; }
void setDay(int new_day) { day = new_day; }
// 其他实用方法,如验证日期是否合法等
// 指定格式打印日期
void printDate() const {
cout << "Date: " << year << "/" << month << "/" << day << endl;
}
// 根据输入字符串解析日期
bool parseString(const string& date_str) {
char delimiter = '/';
size_t pos = 0;
if (!(pos = date_str.find(delimiter))) {
return false;
}
int parsed_year = stoi(date_str.substr(0, pos));
if (parsed_year < 1 || parsed_year > 9999) {
return false;
}
pos += delimiter == '-' ? 1 : 2; // Adjust for possible - instead of /
int parsed_month = stoi(date_str.substr(pos, pos + 2));
if (parsed_month < 1 || parsed_month > 12) {
return false;
}
pos += 2;
int parsed_day = stoi(date_str.substr(pos));
if (parsed_day < 1 || parsed_day > 31) {
return false;
}
setYear(parsed_year);
setMonth(parsed_month);
setDay(parsed_day);
return true;
}
// 其他可能的日期运算方法,例如比较、加减日期等
};
```
阅读全文
相关推荐
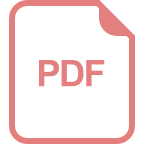
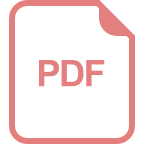
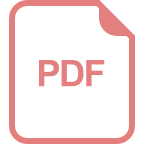














