三维空间 一点为圆中心 求 圆 的点 C# 代码
时间: 2024-04-09 10:33:17 浏览: 21
以下是使用C#编写的代码示例,用于求解三维空间中以给定点为圆心的圆上的点:
```csharp
using System;
public class Point
{
public double X { get; set; }
public double Y { get; set; }
public double Z { get; set; }
public Point(double x, double y, double z)
{
X = x;
Y = y;
Z = z;
}
}
public class Circle
{
public Point Center { get; set; }
public double Radius { get; set; }
public Circle(Point center, double radius)
{
Center = center;
Radius = radius;
}
}
public class Program
{
public static Point[] CalculateCirclePoints(Circle circle, int numPoints)
{
Point[] points = new Point[numPoints];
double angleDelta = 2 * Math.PI / numPoints;
for (int i = 0; i < numPoints; i++)
{
double angle = i * angleDelta;
double x = circle.Radius * Math.Cos(angle) + circle.Center.X;
double y = circle.Radius * Math.Sin(angle) + circle.Center.Y;
double z = circle.Center.Z;
points[i] = new Point(x, y, z);
}
return points;
}
public static void Main(string[] args)
{
Point center = new Point(0, 0, 0);
double radius = 5;
Circle circle = new Circle(center, radius);
int numPoints = 10;
Point[] circlePoints = CalculateCirclePoints(circle, numPoints);
Console.WriteLine("圆上的点:");
foreach (Point point in circlePoints)
{
Console.WriteLine($"({point.X}, {point.Y}, {point.Z})");
}
}
}
```
上述代码定义了一个 `Point` 类表示三维空间中的点,以及一个 `Circle` 类表示圆。`CalculateCirclePoints` 方法用于计算圆上的点,通过给定的圆心和半径,使用极坐标的方式计算圆上的各个点的坐标。在 `Main` 方法中,创建了一个圆,并调用 `CalculateCirclePoints` 方法计算圆上的点。然后将计算得到的点输出到控制台。
相关推荐
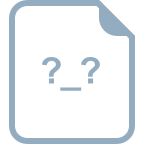














