设计一个测试程序,比较冒泡排序、直接排序、选择排序、箱子排序、堆排序、快速排序及归并排序算法的关键字比较次数和移动次数,待排序表的表长不小于100,表中数据随机产生,至少用5组不同数据作比较,比较指标:关键字参加比较次数和关键字的移动次数(关键字交换记为3次移动),输出比较结果。
时间: 2023-12-20 17:04:52 浏览: 74
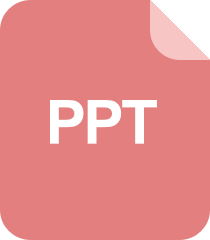
设计一个测试程序比较几种内部排序算法的关键字比较次数和移动次数以取得直观感受。
好的,我会为您设计一个测试程序来比较这些排序算法的关键字比较次数和移动次数。
首先,我们需要生成随机数据,可以使用以下代码:
```python
import random
def generate_data(length):
return [random.randint(0, 1000) for _ in range(length)]
```
接下来,我们可以分别实现这些排序算法,并记录它们的比较次数和移动次数。以下是这些算法的简单实现:
```python
def bubble_sort(arr):
n = len(arr)
comparisons = 0
moves = 0
for i in range(n):
for j in range(n - i - 1):
comparisons += 1
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
moves += 3
return comparisons, moves
def selection_sort(arr):
n = len(arr)
comparisons = 0
moves = 0
for i in range(n):
min_idx = i
for j in range(i + 1, n):
comparisons += 1
if arr[j] < arr[min_idx]:
min_idx = j
arr[i], arr[min_idx] = arr[min_idx], arr[i]
moves += 3
return comparisons, moves
def insertion_sort(arr):
n = len(arr)
comparisons = 0
moves = 0
for i in range(1, n):
key = arr[i]
j = i - 1
while j >= 0 and key < arr[j]:
comparisons += 1
arr[j + 1] = arr[j]
moves += 1
j -= 1
arr[j + 1] = key
moves += 1
return comparisons, moves
def heap_sort(arr):
def heapify(arr, n, i):
nonlocal comparisons, moves
largest = i
l = 2 * i + 1
r = 2 * i + 2
if l < n:
comparisons += 1
if arr[i] < arr[l]:
largest = l
if r < n:
comparisons += 1
if arr[largest] < arr[r]:
largest = r
if largest != i:
arr[i], arr[largest] = arr[largest], arr[i]
moves += 3
heapify(arr, n, largest)
n = len(arr)
comparisons = 0
moves = 0
for i in range(n // 2 - 1, -1, -1):
heapify(arr, n, i)
for i in range(n - 1, 0, -1):
arr[0], arr[i] = arr[i], arr[0]
moves += 3
heapify(arr, i, 0)
return comparisons, moves
def merge_sort(arr):
def merge(arr, l, m, r):
nonlocal comparisons, moves
n1 = m - l + 1
n2 = r - m
L = [0] * n1
R = [0] * n2
for i in range(n1):
L[i] = arr[l + i]
for j in range(n2):
R[j] = arr[m + 1 + j]
i = j = 0
k = l
while i < n1 and j < n2:
comparisons += 1
if L[i] <= R[j]:
arr[k] = L[i]
moves += 1
i += 1
else:
arr[k] = R[j]
moves += 1
j += 1
k += 1
while i < n1:
arr[k] = L[i]
moves += 1
i += 1
k += 1
while j < n2:
arr[k] = R[j]
moves += 1
j += 1
k += 1
def merge_sort_helper(arr, l, r):
nonlocal comparisons, moves
if l < r:
m = (l + r) // 2
merge_sort_helper(arr, l, m)
merge_sort_helper(arr, m + 1, r)
merge(arr, l, m, r)
comparisons = 0
moves = 0
merge_sort_helper(arr, 0, len(arr) - 1)
return comparisons, moves
def quick_sort(arr):
def partition(arr, low, high):
nonlocal comparisons, moves
pivot = arr[high]
i = low - 1
for j in range(low, high):
comparisons += 1
if arr[j] < pivot:
i += 1
arr[i], arr[j] = arr[j], arr[i]
moves += 3
arr[i + 1], arr[high] = arr[high], arr[i + 1]
moves += 3
return i + 1
def quick_sort_helper(arr, low, high):
nonlocal comparisons, moves
if low < high:
pi = partition(arr, low, high)
quick_sort_helper(arr, low, pi - 1)
quick_sort_helper(arr, pi + 1, high)
comparisons = 0
moves = 0
quick_sort_helper(arr, 0, len(arr) - 1)
return comparisons, moves
```
最后,我们可以使用以下代码来测试这些排序算法:
```python
algos = [
("Bubble Sort", bubble_sort),
("Selection Sort", selection_sort),
("Insertion Sort", insertion_sort),
("Heap Sort", heap_sort),
("Merge Sort", merge_sort),
("Quick Sort", quick_sort),
]
lengths = [100, 200, 300, 400, 500]
for length in lengths:
data = generate_data(length)
print(f"Length: {length}")
for name, algo in algos:
comparisons, moves = algo(data.copy())
print(f"{name}: Comparisons = {comparisons}, Moves = {moves}")
print()
```
以上是一个基本的测试程序,它将测试这些排序算法在不同数据集上的比较次数和移动次数,并输出结果。您可以根据需要进行修改和扩展。
阅读全文
相关推荐
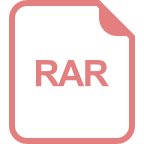

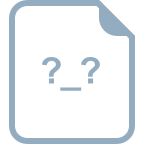
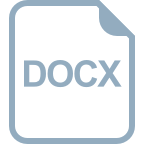
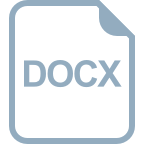
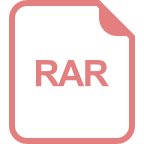
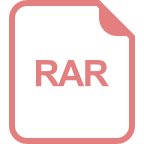
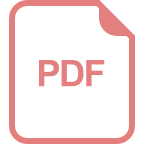
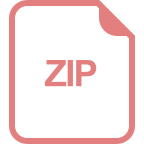
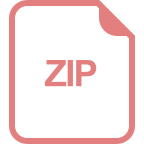
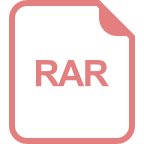
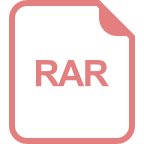
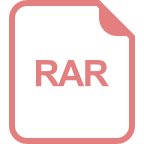
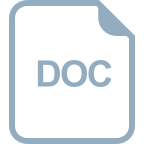
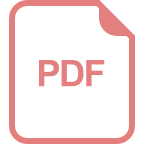
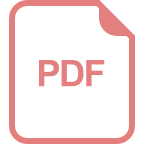