编程题(STL)。首先建立两个int类型的list容器,利用容器的merge算法将其合并,再用容器的sort算法对合并后的容器中数据进行排序。然后设计一个学生类,成员为:学号,姓名,年龄。把5个学生类对象装入vector容器,用sort算法按照年龄大小降序排序,再用find_if算法查找指定的学号的学生信息,最后统计这些学生的年龄之和
时间: 2023-06-18 10:06:59 浏览: 144
下面是代码实现:
```c++
#include <iostream>
#include <list>
#include <vector>
#include <algorithm>
using namespace std;
// 定义学生类
class Student {
public:
int id;
string name;
int age;
Student(int i, string n, int a): id(i), name(n), age(a) {}
};
int main() {
// 定义两个 int 类型的 list 容器并插入数据
list<int> l1 = {5, 3, 2, 4, 1};
list<int> l2 = {6, 8, 7, 9, 10};
// 合并两个容器并排序
l1.merge(l2);
l1.sort();
// 输出合并后的容器
for (auto i : l1) {
cout << i << " ";
}
cout << endl;
// 定义一个存储学生类对象的 vector 容器并插入数据
vector<Student> v = {
Student(1, "Tom", 20),
Student(2, "Jerry", 18),
Student(3, "Alice", 22),
Student(4, "Bob", 19),
Student(5, "Linda", 21)
};
// 按照年龄大小降序排序
sort(v.begin(), v.end(), [](const Student& s1, const Student& s2) {
return s1.age > s2.age;
});
// 输出排序后的学生信息
for (auto s : v) {
cout << "id: " << s.id << ", name: " << s.name << ", age: " << s.age << endl;
}
// 查找指定学号的学生信息
int id = 3;
auto it = find_if(v.begin(), v.end(), [id](const Student& s) {
return s.id == id;
});
if (it != v.end()) {
cout << "Found student with id " << id << ": " << it->name << endl;
} else {
cout << "Student with id " << id << " not found!" << endl;
}
// 统计学生年龄之和
int sum = 0;
for (auto s : v) {
sum += s.age;
}
cout << "Total age: " << sum << endl;
return 0;
}
```
相关推荐
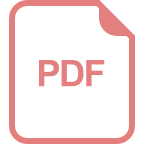
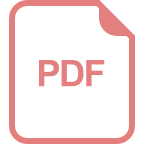
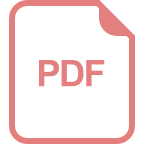














