spritejs实现绘制激光粒子散点
时间: 2023-11-27 12:02:30 浏览: 33
要使用spritejs实现绘制激光粒子散点,你需要在Vue项目中使用spritejs。以下是一个简单的实现步骤:
1. 安装spritejs
你可以使用npm安装spritejs。在终端中运行以下命令:
```
npm install spritejs
```
2. 创建spritejs画布
在Vue组件中,你需要创建一个spritejs画布。以下是一个示例:
```javascript
import spritejs from 'spritejs'
export default {
mounted() {
const container = document.getElementById('container')
this.stage = new spritejs.Stage({
container,
width: container.offsetWidth,
height: container.offsetHeight
})
}
}
```
3. 创建激光粒子散点
要创建激光粒子散点,你需要创建一个spritejs粒子系统。以下是一个示例:
```javascript
import spritejs from 'spritejs'
export default {
mounted() {
const container = document.getElementById('container')
this.stage = new spritejs.Stage({
container,
width: container.offsetWidth,
height: container.offsetHeight
})
// 创建激光粒子散点
const particles = new spritejs.ParticleSystem({
texture: 'path/to/texture.png',
maxSize: 1000,
maxAge: 100,
emitMode: 'once',
life: [1, 2],
pos: [100, 100],
size: [5, 10],
speed: [1, 2],
angle: [0, 360],
rotation: [0, 360],
fillColor: [255, 255, 255, 1],
blendMode: 'lighter',
emitter: {
type: 'circle',
radius: 10
}
})
this.stage.layer().appendChild(particles)
particles.emit()
}
}
```
4. 添加动画效果
要添加动画效果,你需要使用spritejs的动画循环。在Vue组件中,你可以使用requestAnimationFrame方法来实现动画循环。以下是一个示例:
```javascript
import spritejs from 'spritejs'
export default {
mounted() {
const container = document.getElementById('container')
this.stage = new spritejs.Stage({
container,
width: container.offsetWidth,
height: container.offsetHeight
})
// 创建激光粒子散点
const particles = new spritejs.ParticleSystem({
texture: 'path/to/texture.png',
maxSize: 1000,
maxAge: 100,
emitMode: 'once',
life: [1, 2],
pos: [100, 100],
size: [5, 10],
speed: [1, 2],
angle: [0, 360],
rotation: [0, 360],
fillColor: [255, 255, 255, 1],
blendMode: 'lighter',
emitter: {
type: 'circle',
radius: 10
}
})
this.stage.layer().appendChild(particles)
particles.emit()
// 添加动画效果
const animate = () => {
particles.update()
requestAnimationFrame(animate)
}
animate()
}
}
```
这样,你就可以使用spritejs和Vue实现绘制激光粒子散点的动画效果了。
相关推荐
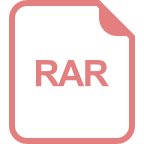














