html,js实现定时切换或者图片轮播效果。在能力范围内添加左右tab切换效果。
时间: 2024-02-20 18:58:58 浏览: 60
以下是一个基本的 HTML、CSS 和 JavaScript 实现定时切换和图片轮播的代码,并且添加了左右 tab 切换效果:
HTML 代码:
```html
<div class="slideshow">
<div class="slideshow-slides">
<div class="slideshow-slide active">
<img src="image1.jpg" alt="Image 1">
</div>
<div class="slideshow-slide">
<img src="image2.jpg" alt="Image 2">
</div>
<div class="slideshow-slide">
<img src="image3.jpg" alt="Image 3">
</div>
</div>
<div class="slideshow-tabs">
<div class="slideshow-tab active"></div>
<div class="slideshow-tab"></div>
<div class="slideshow-tab"></div>
</div>
<a class="slideshow-control prev" href="#">
<span class="slideshow-control-icon">❮</span>
</a>
<a class="slideshow-control next" href="#">
<span class="slideshow-control-icon">❯</span>
</a>
</div>
```
CSS 代码:
```css
.slideshow {
position: relative;
}
.slideshow-slides {
position: relative;
overflow: hidden;
width: 100%;
}
.slideshow-slide {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
opacity: 0;
transition: opacity 0.6s ease-in-out;
}
.slideshow-slide.active {
opacity: 1;
}
.slideshow-tabs {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
display: flex;
}
.slideshow-tab {
width: 10px;
height: 10px;
margin: 0 10px;
border-radius: 50%;
background-color: rgba(0,0,0,0.5);
cursor: pointer;
opacity: 0.5;
transition: opacity 0.2s ease-in-out;
}
.slideshow-tab.active {
opacity: 1;
}
.slideshow-control {
position: absolute;
top: 50%;
transform: translateY(-50%);
z-index: 1;
display: flex;
justify-content: center;
align-items: center;
width: 50px;
height: 50px;
border-radius: 50%;
background-color: rgba(0,0,0,0.5);
color: #fff;
text-decoration: none;
font-size: 30px;
opacity: 0.5;
transition: opacity 0.2s ease-in-out;
}
.slideshow-control:hover {
opacity: 1;
}
.slideshow-control.prev {
left: 0;
}
.slideshow-control.next {
right: 0;
}
```
JavaScript 代码:
```javascript
var slides = document.querySelectorAll('.slideshow-slide');
var tabs = document.querySelectorAll('.slideshow-tab');
var controls = document.querySelectorAll('.slideshow-control');
var currentIndex = 0;
var timeoutId = null;
function goTo(index) {
if (index < 0) {
index = slides.length - 1;
} else if (index >= slides.length) {
index = 0;
}
slides[currentIndex].classList.remove('active');
slides[index].classList.add('active');
tabs[currentIndex].classList.remove('active');
tabs[index].classList.add('active');
currentIndex = index;
if (timeoutId) {
clearTimeout(timeoutId);
timeoutId = null;
}
timeoutId = setTimeout(function() {
goTo(currentIndex + 1);
}, 5000);
}
tabs.forEach(function(tab, index) {
tab.addEventListener('click', function() {
goTo(index);
});
});
controls.forEach(function(control) {
control.addEventListener('click', function(event) {
event.preventDefault();
if (control.classList.contains('prev')) {
goTo(currentIndex - 1);
} else if (control.classList.contains('next')) {
goTo(currentIndex + 1);
}
});
});
goTo(0);
```
这段代码实现了一个简单的定时切换和图片轮播,包括左右切换按钮、 tab 切换和自动播放功能。你可以根据需要修改样式和调整功能。
阅读全文
相关推荐
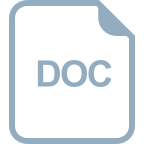
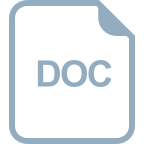
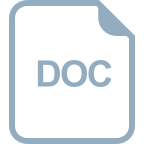
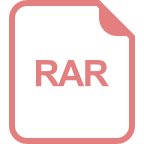
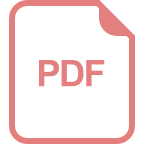
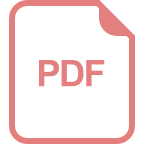
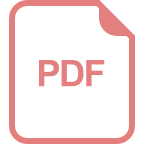
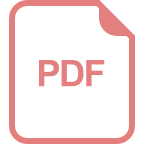
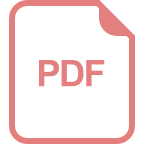
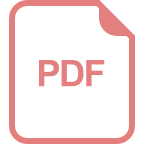
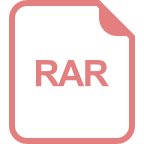
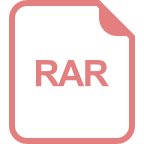
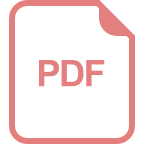
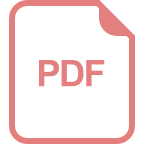
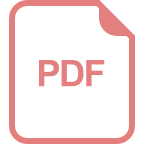
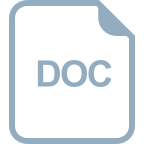
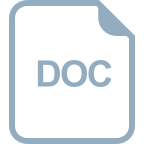