inputstream转为File
时间: 2024-03-06 08:21:06 浏览: 67
要将`InputStream`转为`File`,可以按照以下步骤:
1. 创建一个临时文件,可以使用Java的`File.createTempFile()`方法来创建。
2. 将`InputStream`中的数据写入临时文件中,可以使用Java的`FileOutputStream`来实现。
以下是一个示例代码:
```java
public static File inputStreamToFile(InputStream inputStream) throws IOException {
File tempFile = File.createTempFile("temp", ".tmp");
tempFile.deleteOnExit();
try (FileOutputStream outputStream = new FileOutputStream(tempFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
}
return tempFile;
}
```
这个方法接受一个`InputStream`作为参数,返回一个`File`对象。注意,在方法结束时,临时文件会被删除。
相关问题
inputstream转为File路径
无法将InputStream直接转换为File路径,因为InputStream是从输入源读取数据的流,而File路径是指向文件的路径。在将InputStream转换为File路径之前,需要先从InputStream中读取数据并将其写入到一个临时文件中,然后再获取该临时文件的路径。以下是一种可能的实现方式:
```java
public static String convertInputStreamToFilepath(InputStream inputStream) throws IOException {
File tempFile = File.createTempFile("inputStreamToFile", ".tmp");
try (OutputStream outputStream = new FileOutputStream(tempFile)) {
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
}
return tempFile.getAbsolutePath();
}
```
上述代码将输入流中的数据读取到一个临时文件中,然后返回该临时文件的绝对路径。需要注意的是,使用完临时文件后应该将其删除,以释放磁盘空间。
java inputstream 转为file对象
可以使用以下代码将 Java InputStream 转换为 File 对象:
```java
public static File inputStreamToFile(InputStream inputStream, String filePath) throws IOException {
File file = new File(filePath);
OutputStream outputStream = new FileOutputStream(file);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
outputStream.close();
inputStream.close();
return file;
}
```
其中,inputStream 是要转换的 InputStream 对象,filePath 是要保存的文件路径。这个方法会将 InputStream 中的数据写入到指定的文件中,并返回 File 对象。
阅读全文
相关推荐









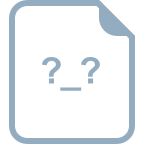






