用C语言输入 多组数据,每组数据有两行。第一行为一个整数n,n表示入队序列A的长度(n个数依次连续入队,中间没有出队的情况),第二行为序列A(空格分隔的n个整数)。当n=0时,输入结束。 输出 对应每组数据输出一行。依次输出队列中所有的整数,每两个整数之间用空格分隔。
时间: 2024-05-07 15:16:25 浏览: 126
这是一道关于队列的问题,可以使用C语言中的队列数据结构来解决。以下是代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 1000
int queue[MAX_SIZE];
int front = 0, rear = 0;
void enqueue(int value) {
if (rear >= MAX_SIZE) {
printf("Queue is full.\n");
exit(1);
}
queue[rear++] = value;
}
int dequeue() {
if (front == rear) {
printf("Queue is empty.\n");
exit(1);
}
return queue[front++];
}
int main() {
int n;
while (scanf("%d", &n) != EOF && n != 0) {
front = rear = 0;
for (int i = 0; i < n; i++) {
int value;
scanf("%d", &value);
enqueue(value);
}
while (front < rear) {
int value = dequeue();
printf("%d", value);
if (front < rear) {
printf(" ");
}
}
printf("\n");
}
return 0;
}
```
当输入一组数据后,程序会将所有整数依次入队,并在队列中依次出队并输出。当输入n=0时,程序结束。
阅读全文
相关推荐
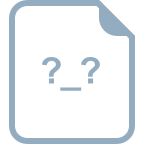
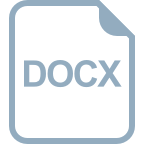





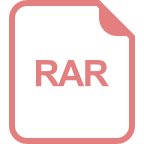
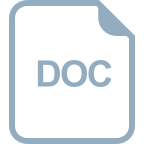
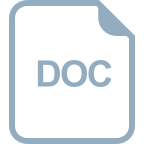
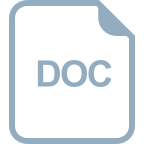
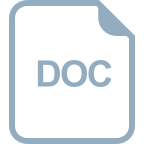
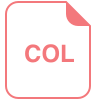
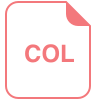
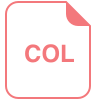
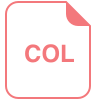