HBuilder用vue开发一个页面,实现登陆功能
时间: 2024-03-08 13:48:06 浏览: 25
好的,以下是步骤:
1. 在HBuilder中创建一个Vue项目,可以选择使用Vue-cli或手动创建。如果使用Vue-cli,可以在终端中运行以下命令:
```
vue create your-project-name
```
2. 创建完成后,在HBuilder的项目管理器中找到src目录,其中的App.vue文件是Vue项目的入口文件。
3. 在App.vue文件中,添加一个登录表单,包括用户名和密码输入框,以及登录按钮:
```
<template>
<div>
<h1>登录</h1>
<form>
<div>
<label>用户名:</label>
<input type="text" v-model="username">
</div>
<div>
<label>密码:</label>
<input type="password" v-model="password">
</div>
<button @click.prevent="login">登录</button>
</form>
</div>
</template>
<script>
export default {
name: 'App',
data() {
return {
username: '',
password: ''
}
},
methods: {
login() {
// 在这里编写登录逻辑
}
}
}
</script>
```
4. 在methods中添加login方法,用于处理登录逻辑。这里我们可以使用axios库发送POST请求,将用户名和密码发送到后端进行验证。如果验证通过,则跳转到主页。
```
<template>
<div>
<h1>登录</h1>
<form>
<div>
<label>用户名:</label>
<input type="text" v-model="username">
</div>
<div>
<label>密码:</label>
<input type="password" v-model="password">
</div>
<button @click.prevent="login">登录</button>
</form>
</div>
</template>
<script>
import axios from 'axios'
export default {
name: 'App',
data() {
return {
username: '',
password: ''
}
},
methods: {
login() {
axios.post('/api/login', {
username: this.username,
password: this.password
}).then(response => {
if (response.data.success) {
// 登录成功,跳转到主页
this.$router.push('/home')
} else {
// 登录失败,提示错误信息
alert(response.data.message)
}
}).catch(error => {
// 请求失败,提示错误信息
alert(error.message)
})
}
}
}
</script>
```
5. 在后端接口中,编写对应的登录验证逻辑,并返回登录结果。在这里我们假设后端接口为/api/login,返回格式为JSON,包括success和message两个字段。
```
router.post('/api/login', (req, res) => {
const { username, password } = req.body
if (username === 'admin' && password === '123456') {
res.json({ success: true })
} else {
res.json({ success: false, message: '用户名或密码错误' })
}
})
```
6. 最后,启动后端服务器,并在HBuilder中运行到浏览器中,即可测试登录功能。
以上是简单的示例,具体的登录功能实现可以根据自己的需求进行设计和编写。
相关推荐
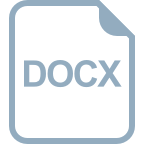
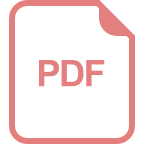














