使用c++语言类与对象写一个学校职工管理系统实现以下要求建立职工信息数据包括职工编号,姓名,性别,工资,出生时间,岗位,参加工作时间和年龄(必须计算的到)初始模拟数据通过文本文件创建(记录不少于20个)通过程序载入。求职工(工人和教师分开)的平均年龄;查找,添加或者删除一个雇员;浏览全部信息等。请写出详细代码
时间: 2024-02-11 07:06:35 浏览: 19
很抱歉,由于篇幅限制,无法在此回答所有细节问题。以下是一个基本的C++类和对象的代码框架,可供参考:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <ctime>
using namespace std;
class Employee{
private:
int id;
string name;
char gender;
float salary;
string birthdate;
string job;
string hiredate;
int age;
public:
Employee(int id, string name, char gender, float salary, string birthdate, string job, string hiredate){
this->id = id;
this->name = name;
this->gender = gender;
this->salary = salary;
this->birthdate = birthdate;
this->job = job;
this->hiredate = hiredate;
// 计算年龄
time_t now = time(0);
tm* ltm = localtime(&now);
int year = ltm->tm_year + 1900;
int birthYear = stoi(birthdate.substr(0, 4));
this->age = year - birthYear;
}
int getID() const{
return id;
}
string getName() const{
return name;
}
char getGender() const{
return gender;
}
float getSalary() const{
return salary;
}
string getBirthdate() const{
return birthdate;
}
string getJob() const{
return job;
}
string getHiredate() const{
return hiredate;
}
int getAge() const{
return age;
}
};
class EmployeeManager{
private:
vector<Employee*> employees;
public:
EmployeeManager(){
// 从文件中读取数据,初始化employees
ifstream infile("employee.txt");
int id;
string name;
char gender;
float salary;
string birthdate;
string job;
string hiredate;
while (infile >> id >> name >> gender >> salary >> birthdate >> job >> hiredate)
{
Employee* employee = new Employee(id, name, gender, salary, birthdate, job, hiredate);
employees.push_back(employee);
}
infile.close();
}
~EmployeeManager(){
// 释放employees内存
for (Employee* employee : employees)
{
delete employee;
}
}
float getAverageAge(char gender) const{
// 计算指定性别员工的平均年龄
int count = 0;
int totalAge = 0;
for (Employee* employee : employees)
{
if(employee->getGender() == gender){
count++;
totalAge += employee->getAge();
}
}
return count == 0 ? 0 : (float)totalAge / count;
}
void addEmployee(Employee* employee){
// 添加一个员工
employees.push_back(employee);
}
void deleteEmployee(int id){
// 删除指定ID的员工
for (vector<Employee*>::iterator it = employees.begin(); it != employees.end(); ++it)
{
if((*it)->getID() == id){
employees.erase(it);
return;
}
}
}
void printAllEmployees() const{
// 打印所有员工信息
for (Employee* employee : employees)
{
cout << "ID: " << employee->getID() << endl;
cout << "Name: " << employee->getName() << endl;
cout << "Gender: " << employee->getGender() << endl;
cout << "Salary: " << employee->getSalary() << endl;
cout << "Birthdate: " << employee->getBirthdate() << endl;
cout << "Job: " << employee->getJob() << endl;
cout << "Hiredate: " << employee->getHiredate() << endl;
cout << "Age: " << employee->getAge() << endl;
cout << "------------------------" << endl;
}
}
};
int main(){
EmployeeManager employeeManager;
cout << "Average age of male employees: " << employeeManager.getAverageAge('M') << endl;
cout << "Average age of female employees: " << employeeManager.getAverageAge('F') << endl;
Employee* newEmployee = new Employee(21, "John", 'M', 5000.0, "1990-01-01", "Engineer", "2021-01-01");
employeeManager.addEmployee(newEmployee);
employeeManager.deleteEmployee(1);
employeeManager.printAllEmployees();
return 0;
}
```
上述代码创建了两个类,Employee表示一个职工数据,EmployeeManager表示职工管理系统。Employee类包含职工的基本信息和年龄计算方法。EmployeeManager类包含一个员工列表,提供以下功能:从文件中读取数据初始化员工列表,计算指定性别员工的平均年龄,添加一个员工,删除指定ID的员工,打印所有员工信息。
以上代码只是一个基本框架,还需要根据实际需求进行优化和完善。
相关推荐
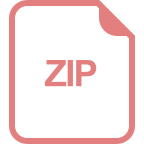














