设计一个小型通讯录管理程序,实现通讯录的新建、保存、以及通讯录的增、 删、改、查功能。(提示:通过链表进行通讯录各种操作,并使用文件存储通讯录。)
时间: 2023-07-10 08:15:35 浏览: 65
好的,我来为您提供一个简单的通讯录管理程序的设计思路和代码实现。这个程序将使用链表数据结构来存储通讯录信息,并通过文件来保存和读取通讯录数据。
首先,我们需要定义一个通讯录项的结构体,包含姓名、电话号码和地址等基本信息:
```c
typedef struct {
char name[50];
char phone[20];
char address[100];
} Contact;
```
接下来,我们需要定义一个链表节点的结构体,它包含一个指向通讯录项的指针和一个指向下一个节点的指针:
```c
typedef struct _Node {
Contact *contact;
struct _Node *next;
} Node;
```
然后,我们需要定义一个链表的结构体,包含指向链表头节点的指针和链表中节点的数量:
```c
typedef struct {
Node *head;
int count;
} LinkedList;
```
接下来,我们可以实现通讯录的各种操作:
1. 创建一个新的通讯录
```c
LinkedList *createLinkedList() {
LinkedList *list = (LinkedList *)malloc(sizeof(LinkedList));
list->head = NULL;
list->count = 0;
return list;
}
```
2. 添加一个新的通讯录项
```c
void addContact(LinkedList *list, Contact *contact) {
Node *node = (Node *)malloc(sizeof(Node));
node->contact = contact;
node->next = list->head;
list->head = node;
list->count++;
}
```
3. 删除一个通讯录项
```c
void removeContact(LinkedList *list, int index) {
if (index < 0 || index >= list->count) {
printf("Error: invalid index\n");
return;
}
Node *prev = NULL;
Node *current = list->head;
for (int i = 0; i < index; i++) {
prev = current;
current = current->next;
}
if (prev != NULL) {
prev->next = current->next;
} else {
list->head = current->next;
}
free(current->contact);
free(current);
list->count--;
}
```
4. 修改一个通讯录项
```c
void editContact(LinkedList *list, int index, Contact *newContact) {
if (index < 0 || index >= list->count) {
printf("Error: invalid index\n");
return;
}
Node *node = list->head;
for (int i = 0; i < index; i++) {
node = node->next;
}
free(node->contact);
node->contact = newContact;
}
```
5. 查找一个通讯录项
```c
int findContact(LinkedList *list, char *name) {
Node *node = list->head;
int index = 0;
while (node != NULL) {
if (strcmp(node->contact->name, name) == 0) {
return index;
}
node = node->next;
index++;
}
return -1;
}
```
6. 保存通讯录到文件
```c
void saveContactsToFile(LinkedList *list, char *filename) {
FILE *fp = fopen(filename, "w");
if (fp == NULL) {
printf("Error: cannot open file %s\n", filename);
return;
}
Node *node = list->head;
while (node != NULL) {
fprintf(fp, "%s,%s,%s\n", node->contact->name, node->contact->phone, node->contact->address);
node = node->next;
}
fclose(fp);
}
```
7. 从文件中读取通讯录
```c
void loadContactsFromFile(LinkedList *list, char *filename) {
FILE *fp = fopen(filename, "r");
if (fp == NULL) {
printf("Error: cannot open file %s\n", filename);
return;
}
char line[256];
while (fgets(line, sizeof(line), fp) != NULL) {
char name[50], phone[20], address[100];
sscanf(line, "%[^,],%[^,],%[^\n]", name, phone, address);
Contact *contact = (Contact *)malloc(sizeof(Contact));
strncpy(contact->name, name, sizeof(contact->name));
strncpy(contact->phone, phone, sizeof(contact->phone));
strncpy(contact->address, address, sizeof(contact->address));
addContact(list, contact);
}
fclose(fp);
}
```
这样,我们就完成了一个简单的通讯录管理程序。您可以根据自己的需要对代码进行修改和扩展。
相关推荐
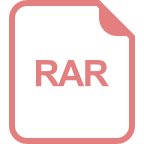
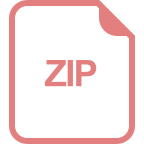














