写出一个可以增加,删减,查找,创建,修改的单链表源代码
时间: 2023-04-09 12:00:25 浏览: 81
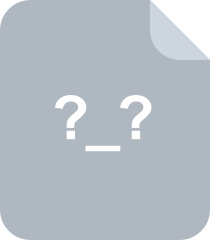
单链表源码,包括增删改查
以下是单链表的源代码,可以实现增加、删除、查找、创建和修改操作:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createList() {
Node *head = (Node *)malloc(sizeof(Node));
head->next = NULL;
return head;
}
void insert(Node *head, int data) {
Node *p = (Node *)malloc(sizeof(Node));
p->data = data;
p->next = head->next;
head->next = p;
}
void delete(Node *head, int data) {
Node *p = head->next;
Node *pre = head;
while (p != NULL) {
if (p->data == data) {
pre->next = p->next;
free(p);
return;
}
pre = p;
p = p->next;
}
}
Node *search(Node *head, int data) {
Node *p = head->next;
while (p != NULL) {
if (p->data == data) {
return p;
}
p = p->next;
}
return NULL;
}
void modify(Node *head, int oldData, int newData) {
Node *p = search(head, oldData);
if (p != NULL) {
p->data = newData;
}
}
void printList(Node *head) {
Node *p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
int main() {
Node *head = createList();
insert(head, 1);
insert(head, 2);
insert(head, 3);
printList(head);
delete(head, 2);
printList(head);
modify(head, 3, 4);
printList(head);
return 0;
}
```
注意:这只是一个简单的单链表实现,可能存在一些问题,比如没有考虑内存泄漏等情况。在实际使用中,需要根据具体情况进行修改和完善。
阅读全文
相关推荐
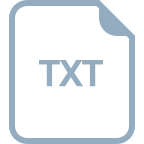
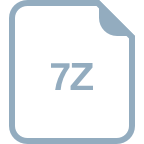
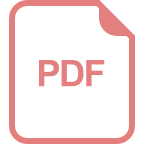
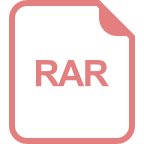
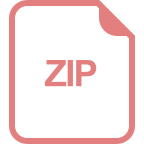
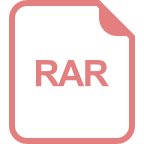
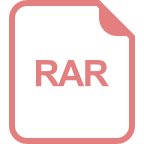
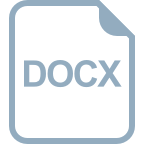
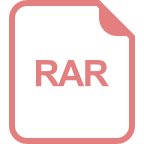
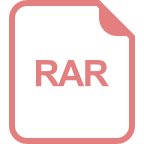






