Pyside6 QGraphicsView实现鼠标滚轮放大缩小 移动
时间: 2023-10-29 11:15:11 浏览: 532
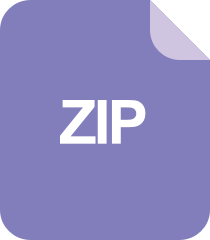
QGraphicsView以鼠标点实现放大缩小、拖拽
使用 PySide6 中的 QGraphicsView 类可以实现鼠标滚轮放大缩小和移动功能。具体实现步骤如下:
1. 继承 QGraphicsView 类创建自定义视图类,例如 MyGraphicsView。
```python
from PySide6.QtWidgets import QGraphicsView
class MyGraphicsView(QGraphicsView):
pass
```
2. 在自定义视图类中重写 wheelEvent() 函数,实现鼠标滚轮缩放功能。可以通过调整视图的缩放比例和滚轮事件的 delta() 值来实现缩放效果。
```python
from PySide6.QtCore import Qt
class MyGraphicsView(QGraphicsView):
def wheelEvent(self, event):
# 改变视图缩放比例
zoomInFactor = 1.25
zoomOutFactor = 1 / zoomInFactor
if event.angleDelta().y() > 0:
zoomFactor = zoomInFactor
else:
zoomFactor = zoomOutFactor
self.scale(zoomFactor, zoomFactor)
```
3. 在自定义视图类中添加 mousePressEvent() 和 mouseMoveEvent() 函数,实现鼠标移动功能。通过记录鼠标按下时的位置和移动后的位置,计算出偏移量并调整视图的位置实现移动效果。
```python
class MyGraphicsView(QGraphicsView):
def __init__(self, parent=None):
super().__init__(parent)
self.setDragMode(QGraphicsView.ScrollHandDrag) # 设置拖拽模式为滚动条拖拽
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self._lastPos = event.pos()
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
delta = event.pos() - self._lastPos
self._lastPos = event.pos()
self.horizontalScrollBar().setValue(self.horizontalScrollBar().value() - delta.x())
self.verticalScrollBar().setValue(self.verticalScrollBar().value() - delta.y())
```
最终实现的 MyGraphicsView 类可以同时实现鼠标滚轮放大缩小和移动功能。
完整代码如下:
```python
from PySide6.QtWidgets import QGraphicsView
from PySide6.QtCore import Qt
class MyGraphicsView(QGraphicsView):
def __init__(self, parent=None):
super().__init__(parent)
self.setDragMode(QGraphicsView.ScrollHandDrag)
def wheelEvent(self, event):
zoomInFactor = 1.25
zoomOutFactor = 1 / zoomInFactor
if event.angleDelta().y() > 0:
zoomFactor = zoomInFactor
else:
zoomFactor = zoomOutFactor
self.scale(zoomFactor, zoomFactor)
def mousePressEvent(self, event):
if event.button() == Qt.LeftButton:
self._lastPos = event.pos()
def mouseMoveEvent(self, event):
if event.buttons() == Qt.LeftButton:
delta = event.pos() - self._lastPos
self._lastPos = event.pos()
self.horizontalScrollBar().setValue(self.horizontalScrollBar().value() - delta.x())
self.verticalScrollBar().setValue(self.verticalScrollBar().value() - delta.y())
```
可以在 PySide6 应用程序中使用 MyGraphicsView 类来创建带有鼠标滚轮放大缩小和移动功能的视图。
阅读全文
相关推荐
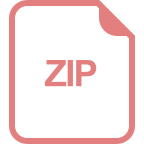

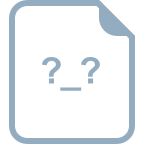













