import package java_java初学者,如何理解package和import?
时间: 2023-12-25 16:04:15 浏览: 167
在Java中,package是一种组织代码的方式,可以将相关的类放在同一个包中。package语句必须在每个Java源文件的开头,用来指定该文件中的类所属的包。例如:
```
package com.example;
public class MyClass {
// class body
}
```
这个例子中,MyClass类属于com.example包。
而import语句则是用来在Java源文件中引入其他包中的类、接口或者静态成员。在使用其他包中的类时,需要使用完整的类名或者使用import语句引入该类。例如:
```
import java.util.ArrayList;
public class MyClass {
public static void main(String[] args) {
ArrayList<String> list = new ArrayList<>();
// code using ArrayList
}
}
```
这个例子中,使用了java.util包中的ArrayList类,通过import语句引入该类。这样就可以直接使用ArrayList类而不需要使用完整的类名java.util.ArrayList。
相关问题
给我下面的代码多写点注释,能让初学者理解:package Pingdemo; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class Ping { public static void main(String[] args) { BufferedReader reader = null; String ipAddress; try { reader = new BufferedReader(new InputStreamReader(System.in)); System.out.println("Please enter IP address: "); ipAddress = reader.readLine(); // 运行ping命令 Process p = Runtime.getRuntime().exec("ping " + ipAddress); // 读取命令的输出 BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream())); String line; while ((line = input.readLine()) != null) { System.out.println(line); } input.close(); } catch (IOException e) { e.printStackTrace(); } finally { if (reader != null) { try { reader.close(); } catch (IOException e) { e.printStackTrace(); } } } } }
这段代码是一个简单的Java程序,它会让用户输入一个IP地址,然后通过运行系统的ping命令,测试与该IP地址的连接状况,并输出ping命令的结果。
注释如下:
```java
package Pingdemo;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Ping {
public static void main(String[] args) {
BufferedReader reader = null; // 用于读取用户输入的IP地址
String ipAddress;
try {
reader = new BufferedReader(new InputStreamReader(System.in)); // 创建输入流对象
System.out.println("Please enter IP address: "); // 提示用户输入IP地址
ipAddress = reader.readLine(); // 读取用户输入的IP地址
// 运行ping命令
Process p = Runtime.getRuntime().exec("ping " + ipAddress);
// 读取命令的输出
BufferedReader input = new BufferedReader(new InputStreamReader(p.getInputStream()));
String line;
while ((line = input.readLine()) != null) { // 逐行读取ping命令的输出
System.out.println(line); // 输出每一行ping命令的结果
}
input.close(); // 关闭输入流
} catch (IOException e) { // 处理可能出现的异常
e.printStackTrace();
} finally { // 无论是否出现异常,都要执行以下代码
if (reader != null) { // 关闭输入流对象
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
阅读全文
相关推荐
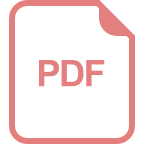
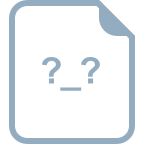
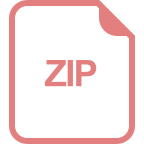
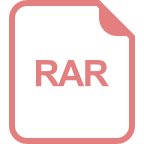
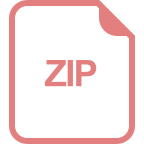
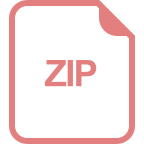
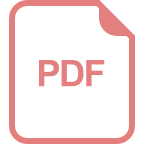
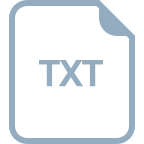
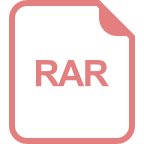
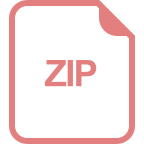
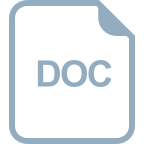
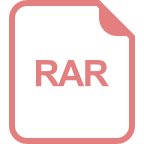
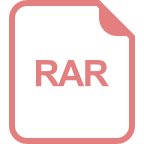