Android 实现NFC模拟卡
时间: 2023-11-11 15:11:30 浏览: 172
要实现Android的NFC模拟卡功能,可以按照以下步骤进行:
1. 在AndroidManifest.xml文件中添加NFC权限:
```
<uses-permission android:name="android.permission.NFC" />
```
2. 创建一个继承HostApduService的类来处理APDU命令:
```
public class MyHostApduService extends HostApduService {
private static final String TAG = "MyHostApduService";
@Override
public byte[] processCommandApdu(byte[] commandApdu, Bundle extras) {
// 处理APDU命令
...
// 返回响应数据
return responseApdu;
}
@Override
public void onDeactivated(int reason) {
Log.d(TAG, "onDeactivated: " + reason);
}
}
```
3. 在AndroidManifest.xml文件中注册该服务:
```
<application>
<service android:name=".MyHostApduService"
android:exported="true"
android:permission="android.permission.BIND_NFC_SERVICE">
<intent-filter>
<action android:name="android.nfc.cardemulation.action.HOST_APDU_SERVICE" />
</intent-filter>
<meta-data android:name="android.nfc.cardemulation.host_apdu_service"
android:resource="@xml/apduservice" />
</service>
</application>
```
其中,@xml/apduservice是一个XML文件,定义了支持的AID(应用标识符)和对应的服务组件:
```
<?xml version="1.0" encoding="utf-8"?>
<host-apdu-service xmlns:android="http://schemas.android.com/apk/res/android"
android:description="@string/app_name"
android:requireDeviceUnlock="false" >
<aid-group android:description="@string/app_name"
android:category="other">
<aid-filter android:name="F0010203040506" />
</aid-group>
</host-apdu-service>
```
4. 在processCommandApdu方法中处理APDU命令,可以根据命令字节码来判断需要执行的操作。例如,如果收到SELECT AID命令,可以返回应用程序的名称和版本号:
```
private static final byte[] AID = { (byte)0xF0, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06 };
private static final byte[] VERSION = { 0x00, 0x01 };
@Override
public byte[] processCommandApdu(byte[] commandApdu, Bundle extras) {
if (Arrays.equals(commandApdu, SELECT_APDU)) {
return concatenateByteArrays(AID, VERSION);
}
return UNKNOWN_COMMAND_RESPONSE;
}
private byte[] concatenateByteArrays(byte[] a, byte[] b) {
byte[] result = new byte[a.length + b.length];
System.arraycopy(a, 0, result, 0, a.length);
System.arraycopy(b, 0, result, a.length, b.length);
return result;
}
```
这样,当读卡器连接到设备时,设备会自动启动MyHostApduService,并等待命令。如果读卡器发送SELECT AID命令,设备会返回应用程序的名称和版本号。
需要注意的是,NFC模拟卡功能需要设备支持Host Card Emulation(HCE)功能。如果设备不支持HCE,那么就无法使用NFC模拟卡功能。
阅读全文
相关推荐
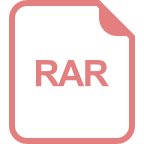

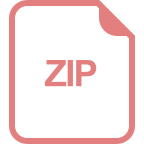
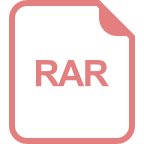
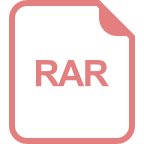
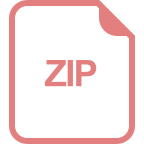
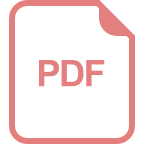
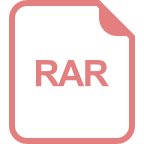
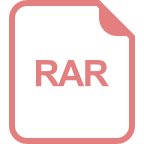
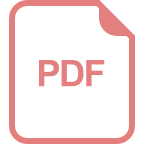
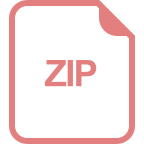
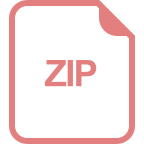
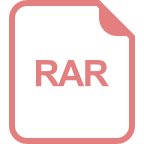
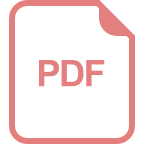
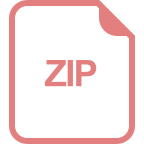