python怎么获取一个文件夹里面图片的数量
时间: 2024-02-25 07:59:33 浏览: 254
你可以使用Python内置的os和glob模块来获取一个文件夹里面图片的数量。具体步骤如下:
```python
import os
import glob
# 定义文件夹路径
folder_path = '/path/to/folder'
# 使用glob模块获取文件夹内所有图片的路径
img_files = glob.glob(os.path.join(folder_path, '*.jpg'))
# 获取图片数量
num_imgs = len(img_files)
# 输出结果
print('文件夹内共有 {} 张图片。'.format(num_imgs))
```
请将`/path/to/folder`替换成你实际的文件夹路径。上述代码中,使用`glob.glob`函数来获取所有以`.jpg`扩展名结尾的文件,然后使用`len`函数获取文件数量。
相关问题
我现在有两个文件夹,一个文件夹里面有很多张图片,另一个文件夹里面又有一个子文件夹,这个子文件夹里面也有很多张图片,请给出python代码对两个文件夹里面图片的数量进行比较,如果数量不同请告诉我具体是哪张图片的数量不同
以下是一个用Python编写的代码示例,用于比较两个文件夹中图片的数量,并告诉您具体哪张图片的数量不同:
```python
import os
def count_images(folder_path):
count = 0
for file in os.listdir(folder_path):
if file.endswith(".jpg") or file.endswith(".png"):
count += 1
return count
def compare_image_counts(folder1, folder2):
images1 = {file: 0 for file in os.listdir(folder1)}
images2 = {file: 0 for file in os.listdir(folder2)}
for file in images1:
if file.endswith(".jpg") or file.endswith(".png"):
images1[file] = count_images(os.path.join(folder1, file))
for file in images2:
if file.endswith(".jpg") or file.endswith(".png"):
images2[file] = count_images(os.path.join(folder2, file))
different_images = []
for file in images1:
if images1[file] != images2.get(file, 0):
different_images.append(file)
return different_images
folder1_path = "path/to/folder1"
folder2_path = "path/to/folder2"
different_images = compare_image_counts(folder1_path, folder2_path)
if len(different_images) > 0:
print("The following images have different counts:")
for image in different_images:
print(image)
else:
print("The image counts in the two folders are the same.")
```
请将`"path/to/folder1"`和`"path/to/folder2"`替换为您实际的文件夹路径。代码将比较两个文件夹中所有以`.jpg`或`.png`结尾的图片的数量,并输出具有不同数量的图片的文件名。如果两个文件夹中的图片数量相同,则输出相应的消息。
帮我用python写一个遍历文件夹 并判断里面的图片数量
当然可以,请参考以下代码:
```python
import os
def count_images(folder_path):
image_extensions = ['.jpg', '.jpeg', '.png', '.bmp', '.gif'] # 常见的图片格式
image_count = 0
for root, dirs, files in os.walk(folder_path):
for file in files:
if os.path.splitext(file)[1].lower() in image_extensions:
image_count += 1
return image_count
folder_path = 'your_folder_path_here'
image_count = count_images(folder_path)
print('There are', image_count, 'images in folder', folder_path)
```
你只需要将`your_folder_path_here`替换成你要遍历的文件夹路径即可。函数`count_images`会递归遍历指定文件夹下的所有子文件夹,并统计出所有图片文件的数量。
阅读全文
相关推荐



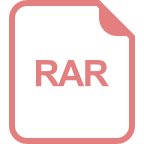









