用python 移动特定文件夹中的文件
时间: 2024-01-31 22:03:39 浏览: 73
你可以使用Python的shutil模块来移动特定文件夹中的文件。以下是一个示例代码,可以将指定路径下的所有txt文件移动到另一个文件夹:
```python
import os
import shutil
# 指定需要移动的文件夹路径
source_folder = "path/to/source/folder"
# 指定需要移动到的文件夹路径
destination_folder = "path/to/destination/folder"
# 获取文件夹中所有文件
files = os.listdir(source_folder)
# 遍历所有文件,移动txt类型的文件到目标文件夹
for file in files:
if file.endswith(".txt"):
shutil.move(os.path.join(source_folder, file), destination_folder)
```
你可以根据自己的需求修改代码,例如修改需要移动的文件类型、指定特定的文件名等。
相关问题
利用python,将文件夹中的文件按照文件名,新建文件夹划分到不同的的文件夹中
在Python中,你可以使用os模块以及shutil模块来实现这个功能。这里有一个基本的示例,假设你有一个源文件夹`source_folder`,你想根据文件名的一些特定模式将其内容分到不同的目标子文件夹里:
```python
import os
import shutil
def move_files_to_subfolders(source_folder, target_folder):
# 遍历源文件夹中的所有文件
for filename in os.listdir(source_folder):
# 检查文件名是否满足某个条件,例如,如果文件名包含 'category_1', 就移动到 'category_1' 文件夹下
if 'category_1' in filename:
category = 'category_1'
elif 'category_2' in filename:
category = 'category_2'
else:
# 如果不匹配任何预设条件,可以选择其他处理方式,比如放到默认分类或其他自定义文件夹
category = 'default'
# 构建目标文件夹路径
subfolder_path = os.path.join(target_folder, category)
# 确保目标目录存在,如果不存在则创建
if not os.path.exists(subfolder_path):
os.makedirs(subfolder_path)
# 移动文件到目标文件夹
source_file = os.path.join(source_folder, filename)
target_file = os.path.join(subfolder_path, filename)
shutil.move(source_file, target_file)
# 调用函数,传入源文件夹和目标根文件夹
move_files_to_subfolders('source_folder', 'target_folder')
```
使用python分类文件夹中不同名称文件
在Python中,你可以使用`os`和`shutil`库来遍历文件夹并按文件名的某种模式分类。这里是一个基本的例子,它将文件分为几个不同的目录,比如按照文件扩展名或文件名的某个前缀:
```python
import os
import shutil
def classify_files(root_dir):
# 定义目标类别目录列表
category_dirs = {
'images': 'Image_Files',
'documents': 'Document_Files',
'.txt': 'Text_Files'
}
for dirpath, dirs, files in os.walk(root_dir): # 遍历文件夹
for file in files:
file_extension = os.path.splitext(file)[1] # 获取文件扩展名
for target_extension, target_dir in category_dirs.items():
if file_extension == target_extension or file.startswith(target_dir):
# 创建或检查目标目录是否存在,然后移动文件
target_path = os.path.join(dirpath, target_dir)
if not os.path.exists(target_path):
os.makedirs(target_path)
source_path = os.path.join(dirpath, file)
destination_path = os.path.join(target_path, file)
shutil.move(source_path, destination_path)
break # 找到匹配的类别后跳出循环
# 调用函数,传入需要分类的根目录路径
classify_files('/path/to/your/directory')
```
在这个例子中,`os.walk()`用于递归地访问文件夹及其内容。对于每个文件,我们检查它的扩展名是否在预定义的类别中,或者如果文件名以特定的前缀开始,则将其归类到相应的目录。
阅读全文
相关推荐
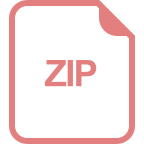

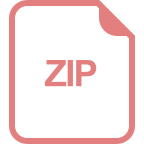
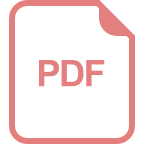
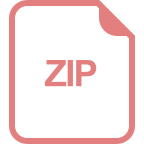
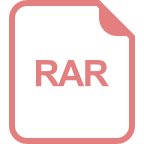
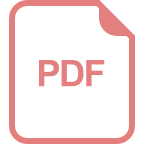
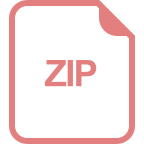
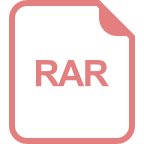
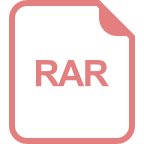
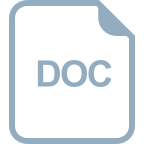
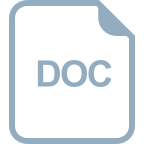
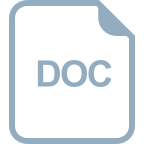