请在flutter中设计蓝牙控制界面,有设备列表,设备查找进度显示,控制按钮,通讯框;并且在app启动时申请蓝牙权限
时间: 2024-02-28 20:52:41 浏览: 136
好的,以下是一个简单的 Flutter 蓝牙控制界面示例,其中包含设备列表,设备查找进度显示,控制按钮和通讯框。同时,在 app 启动时会申请蓝牙权限:
```dart
import 'dart:async';
import 'package:flutter/material.dart';
import 'package:flutter_blue/flutter_blue.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Bluetooth Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new MyHomePage(title: 'Flutter Bluetooth Demo'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
FlutterBlue flutterBlue = FlutterBlue.instance;
StreamSubscription<ScanResult> scanSubscription;
bool isScanning = false;
List<ScanResult> deviceList = [];
BluetoothDevice connectedDevice;
BluetoothCharacteristic characteristic;
TextEditingController controller = new TextEditingController();
List<String> logs = [];
@override
void initState() {
super.initState();
// 请求蓝牙权限
FlutterBlue.instance.requestPermission().then((result) {
if (result == true) {
print("蓝牙权限已授予");
} else {
print("蓝牙权限未授予");
}
});
}
@override
void dispose() {
scanSubscription?.cancel();
super.dispose();
}
void startScan() {
setState(() {
isScanning = true;
deviceList.clear();
});
scanSubscription = flutterBlue.scan().listen((scanResult) {
setState(() {
deviceList.add(scanResult);
});
});
new Timer(const Duration(seconds: 10), () {
stopScan();
});
}
void stopScan() {
scanSubscription?.cancel();
setState(() {
isScanning = false;
});
}
void connectToDevice(BluetoothDevice device) async {
stopScan();
setState(() {
connectedDevice = device;
});
await device.connect();
List<BluetoothService> services = await device.discoverServices();
services.forEach((service) {
service.characteristics.forEach((c) {
if (c.uuid.toString() == "your_characteristic_uuid_here") {
characteristic = c;
}
});
});
setState(() {
logs.add("连接到设备 ${device.name} 成功");
});
}
void disconnectFromDevice() async {
if (connectedDevice != null) {
await connectedDevice.disconnect();
setState(() {
connectedDevice = null;
characteristic = null;
logs.add("已断开连接");
});
}
}
void sendCommand(String command) async {
if (connectedDevice != null && characteristic != null) {
List<int> bytes = utf8.encode(command);
await characteristic.write(bytes);
setState(() {
logs.add("发送命令:$command");
});
}
}
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(title: new Text(widget.title)),
body: new Column(
children: <Widget>[
// 设备列表
new Expanded(
child: new ListView.builder(
itemCount: deviceList.length,
itemBuilder: (BuildContext context, int index) {
ScanResult result = deviceList[index];
return new ListTile(
title: new Text(result.device.name ?? "未知设备"),
subtitle: new Text(result.device.id.toString()),
onTap: () {
connectToDevice(result.device);
},
);
},
),
),
// 设备查找进度显示
new Container(
padding: EdgeInsets.all(16.0),
child: new Row(
children: <Widget>[
new Text(
isScanning ? "正在查找设备..." : "查找已停止",
style: new TextStyle(fontSize: 18.0),
),
new Spacer(),
isScanning
? new CircularProgressIndicator()
: new Container(),
],
),
),
// 控制按钮
new Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: <Widget>[
new RaisedButton(
child: new Text("打开"),
onPressed: () {
sendCommand("open");
},
),
new RaisedButton(
child: new Text("关闭"),
onPressed: () {
sendCommand("close");
},
),
new RaisedButton(
child: new Text("断开连接"),
onPressed: () {
disconnectFromDevice();
},
),
],
),
// 通讯框
new Expanded(
child: new ListView.builder(
itemCount: logs.length,
itemBuilder: (BuildContext context, int index) {
String log = logs[index];
return new ListTile(
title: new Text(log),
);
},
),
),
],
),
floatingActionButton: new FloatingActionButton(
child: isScanning ? new Icon(Icons.stop) : new Icon(Icons.search),
onPressed: () {
if (isScanning) {
stopScan();
} else {
startScan();
}
},
),
);
}
}
```
请注意,上述代码仅用于演示目的,并且需要您根据自己的需求进行修改和优化。此外,您需要在 pubspec.yaml 文件中添加以下依赖项:
```yaml
dependencies:
flutter:
sdk: flutter
flutter_blue: ^0.7.3+1
```
这是使用 FlutterBlue 插件进行蓝牙操作所必需的依赖项。
阅读全文
相关推荐
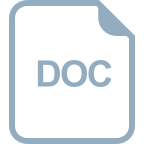
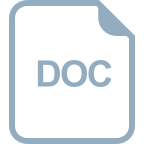
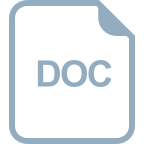
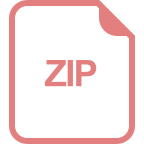
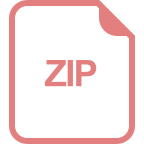
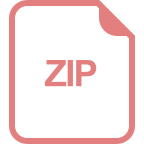
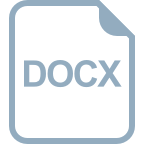
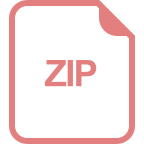
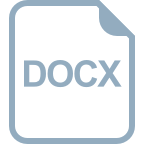
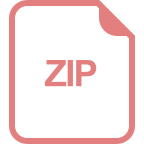
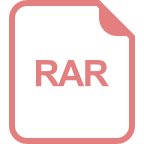
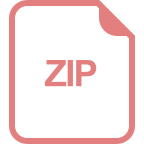
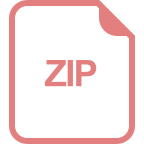
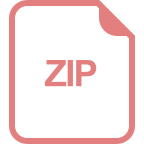
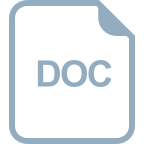
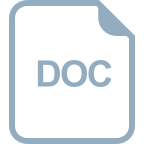
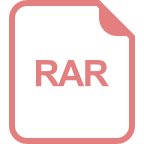
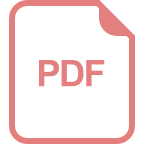