如何将spinner的选中字符与适配器中的餐厅名称进行比较然后更新listview
时间: 2024-06-08 10:07:42 浏览: 68
假设你有一个Spinner来选择餐厅类型,一个ListView来显示餐厅列表,并且ListView的数据源是一个餐厅数组。
首先,你需要创建一个适配器来将餐厅数组显示在ListView上:
```java
public class RestaurantAdapter extends ArrayAdapter<Restaurant> {
public RestaurantAdapter(Context context, ArrayList<Restaurant> restaurants) {
super(context, 0, restaurants);
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
// Inflate the view if needed
if (convertView == null) {
convertView = LayoutInflater.from(getContext()).inflate(R.layout.restaurant_list_item, parent, false);
}
// Get the restaurant at this position
Restaurant restaurant = getItem(position);
// Set the name and other details of the restaurant in the view
TextView nameTextView = convertView.findViewById(R.id.nameTextView);
nameTextView.setText(restaurant.getName());
TextView descriptionTextView = convertView.findViewById(R.id.descriptionTextView);
descriptionTextView.setText(restaurant.getDescription());
// Return the view
return convertView;
}
}
```
然后,在你的Activity中,你需要设置Spinner的适配器和监听器,并且在监听器中更新ListView的数据源和适配器:
```java
public class MainActivity extends AppCompatActivity {
private Spinner mTypeSpinner;
private ListView mRestaurantListView;
private RestaurantAdapter mRestaurantAdapter;
private ArrayList<Restaurant> mRestaurants;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize the views and data
mTypeSpinner = findViewById(R.id.typeSpinner);
mRestaurantListView = findViewById(R.id.restaurantListView);
mRestaurants = new ArrayList<>();
mRestaurants.add(new Restaurant("Pizza Palace", "The best pizza in town!", "Italian"));
mRestaurants.add(new Restaurant("Burger Barn", "Juicy burgers and fries!", "American"));
mRestaurants.add(new Restaurant("Sushi Station", "Fresh sushi rolls made to order!", "Japanese"));
mRestaurantAdapter = new RestaurantAdapter(this, mRestaurants);
mRestaurantListView.setAdapter(mRestaurantAdapter);
// Set the adapter and listener for the type spinner
ArrayAdapter<CharSequence> typeAdapter = ArrayAdapter.createFromResource(this, R.array.restaurant_types, android.R.layout.simple_spinner_item);
typeAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
mTypeSpinner.setAdapter(typeAdapter);
mTypeSpinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
// Get the selected type from the spinner
String selectedType = parent.getItemAtPosition(position).toString();
// Create a new list of restaurants that match the selected type
ArrayList<Restaurant> filteredRestaurants = new ArrayList<>();
for (Restaurant restaurant : mRestaurants) {
if (restaurant.getType().equals(selectedType)) {
filteredRestaurants.add(restaurant);
}
}
// Update the adapter with the filtered list of restaurants
mRestaurantAdapter.clear();
mRestaurantAdapter.addAll(filteredRestaurants);
mRestaurantAdapter.notifyDataSetChanged();
}
@Override
public void onNothingSelected(AdapterView<?> parent) {
// Do nothing
}
});
}
}
```
这里假设你的Spinner的选项是从资源文件中加载的,例如:
```xml
<string-array name="restaurant_types">
<item>Italian</item>
<item>American</item>
<item>Japanese</item>
</string-array>
```
当用户选择Spinner的选项时,监听器会获取选中的字符串,然后遍历餐厅数组,将与选中字符串匹配的餐厅添加到新的ArrayList中。然后清空ListView的适配器并将新的ArrayList添加到适配器中,最后调用notifyDataSetChanged()方法更新ListView的显示。
阅读全文
相关推荐
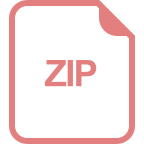
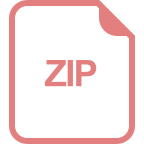

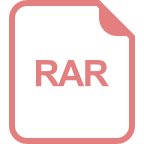
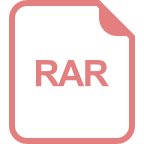
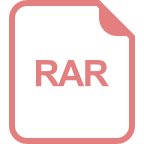
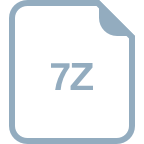
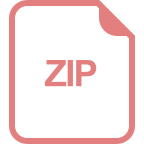
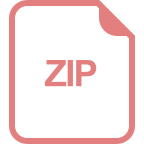
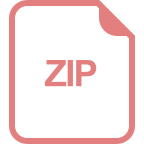
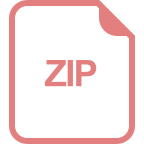
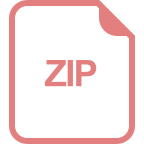
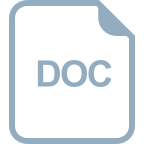
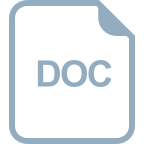
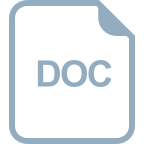
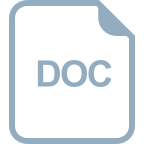
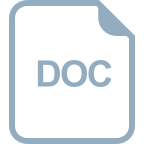
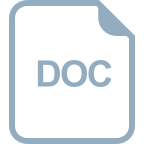
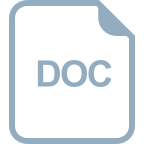