fastapi html 构建一个文件列表,勾选后,点击下载,将列表组成一个组返回给后端,点击上传,将列表组成一个组返回给后端,
时间: 2024-09-06 18:06:35 浏览: 27
FastAPI 是一个现代、快速的 Web 框架,用于构建 API。使用 FastAPI,我们可以轻松地构建一个带有 HTML 界面的文件列表管理应用,实现上传和下载文件的功能。下面是一个基本的实现思路:
1. **构建 HTML 文件列表**:首先,需要创建一个 HTML 页面,列出服务器上可访问的文件。这可以通过 FastAPI 的路由和模板系统(如 Jinja2)来完成。
2. **下载功能**:为了下载文件,你需要为每个文件提供一个下载链接或者按钮。点击下载按钮时,可以使用 HTML 的表单提交或者其他前端技术将文件信息(如文件名)发送到后端。
3. **上传功能**:对于上传功能,你需要在 HTML 中创建一个上传表单,并设置表单的 `enctype` 为 `multipart/form-data`,以便上传文件。点击上传按钮后,表单数据会被提交到后端。
4. **后端处理**:在 FastAPI 后端,你需要定义相应的路由来处理文件列表的获取、文件的下载请求和文件的上传请求。在处理下载请求时,可以通过文件路径返回文件,而在处理上传请求时,可以将接收到的文件保存到服务器。
下面是一个简化的示例代码:
```python
from fastapi import FastAPI, File, UploadFile, HTTPException
from fastapi.templating import Jinja2Templates
from fastapi.staticfiles import StaticFiles
import os
app = FastAPI()
# 设置静态文件目录,用于存放上传的文件
app.mount("/static", StaticFiles(directory="static"), name="static")
# 设置模板目录
templates = Jinja2Templates(directory="templates")
# 文件列表,实际应用中应该是从文件系统动态获取的
file_list = ["file1.txt", "file2.txt", "file3.jpg"]
@app.get("/files/", response_class=HTMLResponse)
async def list_files():
return templates.TemplateResponse("file_list.html", {"file_list": file_list})
@app.post("/upload/")
async def upload_file(file: UploadFile = File(...)):
try:
# 文件保存逻辑
with open(os.path.join("static", file.filename), "wb") as buffer:
buffer.write(await file.read())
return {"filename": file.filename}
except Exception as e:
raise HTTPException(status_code=500, detail="Error saving file") from e
@app.get("/download/{filename}")
async def download_file(filename: str):
file_path = os.path.join("static", filename)
if os.path.exists(file_path):
return FileResponse(path=file_path, filename=filename)
else:
raise HTTPException(status_code=404, detail="File not found")
```
对应的 `file_list.html` 模板可能如下:
```html
<!DOCTYPE html>
<html>
<head>
<title>文件列表</title>
</head>
<body>
<h1>文件列表</h1>
<form action="/upload/" method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<input type="submit" value="上传文件" />
</form>
<ul>
{% for file in file_list %}
<li>
<a href="/download/{{ file }}">下载 {{ file }}</a>
</li>
{% endfor %}
</ul>
</body>
</html>
```
相关推荐
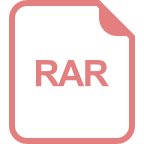
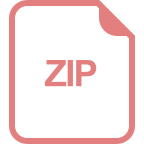
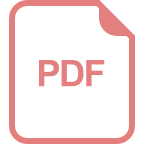













