python中的父类与子类
时间: 2024-05-01 12:19:12 浏览: 87
在 Python 中,类可以继承其他类。我们称被继承的类为父类,继承的类为子类。子类可以使用父类的属性和方法,并且还可以添加自己的属性和方法。
下面是一个简单的例子,其中 `Animal` 是父类,`Dog` 是子类:
```python
class Animal:
def __init__(self, name):
self.name = name
def speak(self):
print("This animal speaks.")
class Dog(Animal):
def __init__(self, name, breed):
super().__init__(name)
self.breed = breed
def speak(self):
print("Woof!")
```
在这个例子中,`Animal` 类有一个属性 `name` 和一个方法 `speak`,`Dog` 类继承了 `Animal` 类,并且添加了一个属性 `breed` 和一个方法 `speak`。`Dog` 类的 `__init__` 方法使用 `super()` 函数调用了父类的构造方法,从而初始化了 `name` 属性。
使用这个类可以这样做:
```python
animal = Animal("generic animal")
dog = Dog("Fido", "Poodle")
print(animal.name) # "generic animal"
animal.speak() # "This animal speaks."
print(dog.name) # "Fido"
print(dog.breed) # "Poodle"
dog.speak() # "Woof!"
```
在这个例子中,`animal` 对象是 `Animal` 类的实例,`dog` 对象是 `Dog` 类的实例。注意到我们调用了 `dog.speak()` 方法,它覆盖了 `Animal` 类的 `speak` 方法。这种行为称为方法重写。
阅读全文
相关推荐
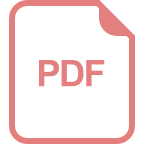



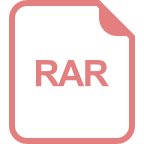











