遗传算法求函数最大值实验.py
时间: 2024-01-16 08:04:01 浏览: 76
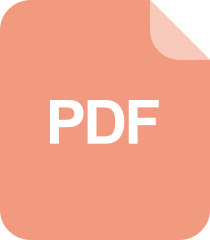
利用遗传算法求解函数最大值.pdf
这是一段使用遗传算法求解函数最大值的 Python 代码,代码中使用了 Python 标准库中的 random 模块。
```
import random
# 目标函数
def target_function(x):
return x**2 - 3*x + 4
# 适应度函数
def fitness_function(x):
return target_function(x)
# 个体编码
def encode_individual():
return random.uniform(-10, 10)
# 初始化种群
def init_population(population_size):
population = []
for i in range(population_size):
population.append(encode_individual())
return population
# 选择操作
def selection(population):
sorted_population = sorted(population, key=lambda x: fitness_function(x), reverse=True)
selected_population = sorted_population[:len(population)//2]
return selected_population
# 交叉操作
def crossover(parent1, parent2):
child1 = (parent1 + parent2) / 2
child2 = (parent1 - parent2) / 2
return child1, child2
# 变异操作
def mutation(child):
mutated_child = child + random.uniform(-0.5, 0.5)
return mutated_child
# 遗传算法主函数
def genetic_algorithm(population_size, max_generations):
population = init_population(population_size)
for i in range(max_generations):
selected_population = selection(population)
new_population = []
while len(new_population) < population_size:
parent1 = random.choice(selected_population)
parent2 = random.choice(selected_population)
child1, child2 = crossover(parent1, parent2)
mutated_child1 = mutation(child1)
mutated_child2 = mutation(child2)
new_population.append(mutated_child1)
new_population.append(mutated_child2)
population = new_population
best_individual = max(population, key=lambda x: fitness_function(x))
return best_individual
# 测试遗传算法
if __name__ == '__main__':
best_individual = genetic_algorithm(population_size=100, max_generations=1000)
best_fitness = fitness_function(best_individual)
print('最优个体:', best_individual)
print('最优适应度:', best_fitness)
```
在这个实验中,我们使用了简单的遗传算法来求解目标函数 $f(x) = x^2 - 3x + 4$ 的最大值。首先,我们定义了目标函数和适应度函数,其中目标函数就是要求解的函数,适应度函数就是根据目标函数计算个体适应度的函数。然后,我们定义了个体编码函数,这里采用了实数编码,即每个个体是一个实数。接着,我们初始化了种群,并定义了选择、交叉和变异等遗传算法的操作。最后,我们使用遗传算法进行求解,并输出了最优个体和最优适应度。
阅读全文
相关推荐
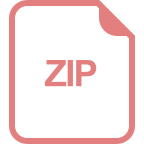
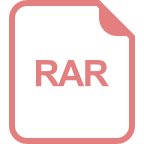
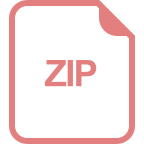
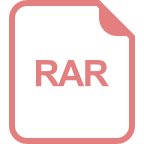
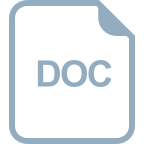
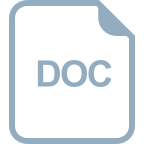
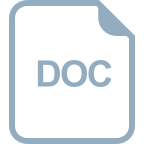
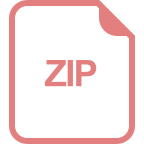
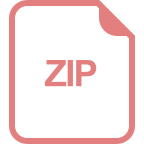
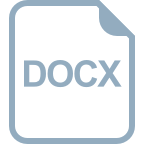
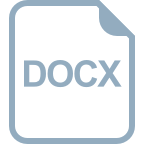
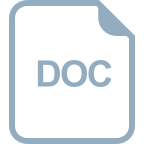
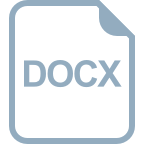
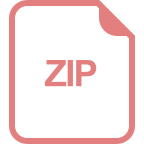
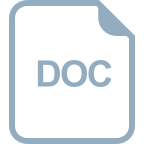