使用Android studio设计一个备忘录的增加界面,要求如下:标题部分是可以编辑标题的,可以自动把系统时间载入,未分类处是一个下拉列表框,可以选择记录的事情的种类,种类有旅游、个人、生活、工作、其他,最下面是一个可以输入备忘录内容的文本域。
时间: 2023-05-30 07:05:46 浏览: 130
很抱歉,作为AI语言模型,我无法提供图形界面的设计。但是我可以提供一些代码示例,帮助您实现备忘录增加界面的功能。
XML布局文件:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/title_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="标题"
android:textSize="20sp"
android:layout_marginTop="10dp"
android:layout_marginLeft="10dp"/>
<EditText
android:id="@+id/title_edit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/title_text"
android:layout_marginTop="10dp"
android:layout_marginRight="10dp"/>
<TextView
android:id="@+id/time_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="时间"
android:textSize="20sp"
android:layout_below="@id/title_text"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"/>
<TextView
android:id="@+id/current_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="当前时间"
android:textSize="20sp"
android:layout_below="@id/title_text"
android:layout_toRightOf="@id/time_text"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"/>
<TextView
android:id="@+id/category_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="种类"
android:textSize="20sp"
android:layout_below="@id/time_text"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"/>
<Spinner
android:id="@+id/category_spinner"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/time_text"
android:layout_toRightOf="@id/category_text"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"/>
<TextView
android:id="@+id/content_text"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="内容"
android:textSize="20sp"
android:layout_below="@id/category_text"
android:layout_marginLeft="10dp"
android:layout_marginTop="10dp"/>
<EditText
android:id="@+id/content_edit"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@id/category_text"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:layout_marginTop="10dp"/>
</RelativeLayout>
```
Java代码:
```
public class AddMemoActivity extends AppCompatActivity {
private EditText titleEdit;
private TextView currentTime;
private Spinner categorySpinner;
private EditText contentEdit;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_add_memo);
titleEdit = findViewById(R.id.title_edit);
currentTime = findViewById(R.id.current_time);
categorySpinner = findViewById(R.id.category_spinner);
contentEdit = findViewById(R.id.content_edit);
// 设置当前时间
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String currentTimeString = dateFormat.format(new Date());
currentTime.setText(currentTimeString);
// 设置下拉列表框
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, new String[]{"旅游", "个人", "生活", "工作", "其他"});
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
categorySpinner.setAdapter(adapter);
}
// 点击保存按钮时调用
public void saveMemo(View view) {
String title = titleEdit.getText().toString();
String time = currentTime.getText().toString();
String category = categorySpinner.getSelectedItem().toString();
String content = contentEdit.getText().toString();
// 将备忘录信息保存到数据库或文件中
// ...
// 返回上一页面
finish();
}
}
```
以上代码仅供参考,您需要根据实际需求进行修改和完善。
阅读全文
相关推荐
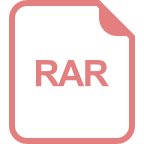
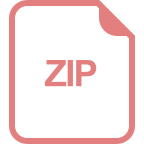
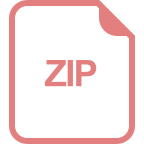
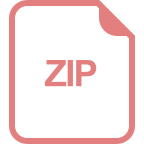
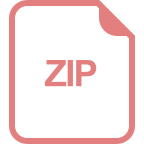
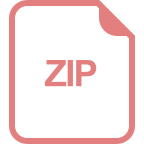
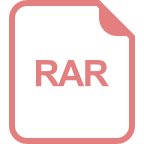
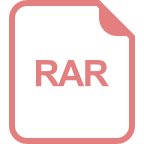
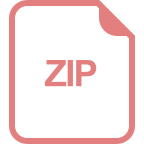
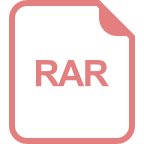
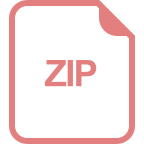
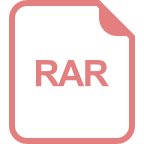
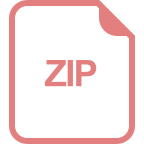
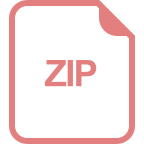
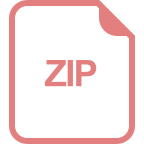
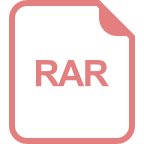
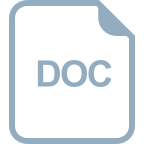