if I have 2 yaml file(you can write whatever yaml files as you like, as long as it can be compiled and run successfully), can you write a python program to compare the difference between these 2 yaml files? The requirement is that if these 2 yaml files are identical, the python program should print out: these 2 yaml files are identical, if these 2 yaml files are not identical, the python program should print out the difference between these 2 yaml files.
时间: 2024-03-27 10:35:10 浏览: 383
Sure, I can help you with that. You can use the PyYAML library to load the YAML files and then compare them using the `deepdiff` library. Here is a sample Python program that implements this functionality:
```python
import yaml
from deepdiff import DeepDiff
def compare_yaml_files(file1, file2):
with open(file1, 'r') as f1, open(file2, 'r') as f2:
data1 = yaml.safe_load(f1)
data2 = yaml.safe_load(f2)
diff = DeepDiff(data1, data2, ignore_order=True)
if not diff:
print("These 2 YAML files are identical")
else:
print("Difference between these 2 YAML files:")
print(diff)
# Example usage
compare_yaml_files('file1.yaml', 'file2.yaml')
```
In this program, the `compare_yaml_files` function takes in the paths to the two YAML files as input parameters. The `yaml.safe_load` function is used to load the YAML data from the files into Python dictionaries. The `deepdiff.DeepDiff` function is then used to compare the two dictionaries and create a diff object. If the diff object is empty, the program prints out that the two YAML files are identical. Otherwise, it prints out the difference between the two files. Note that the `ignore_order=True` argument is used to ignore differences in the order of elements in lists or dictionaries.
阅读全文
相关推荐
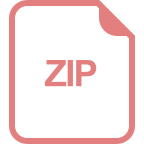
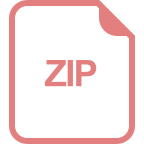
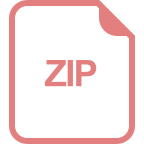
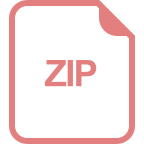
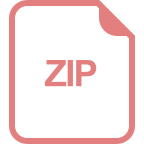
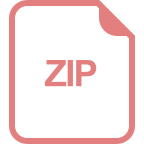
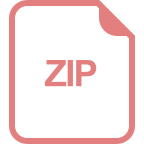
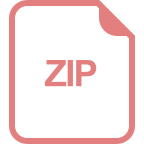
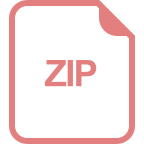
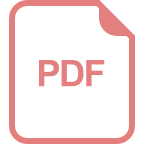
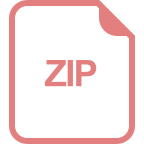
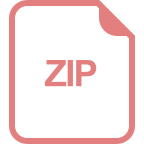