java opensaml
时间: 2023-10-18 13:55:45 浏览: 257
Java OpenSAML是一个用于处理SAML协议的Java库。SAML(Security Assertion Markup Language)是一种用于在不同的安全域之间传递身份认证和授权数据的协议。Java OpenSAML可以帮助开发人员实现SAML协议在Java应用程序中的集成,以实现安全的单点登录和跨域身份验证等功能。
Java OpenSAML提供了一组API,可以用来生成、解析和验证SAML消息。其中,opensaml-core提供了SAML基础结构的实现,opensaml-saml-api提供了SAML核心API的定义,opensaml-saml-impl提供了SAML核心API的实现。
开发人员可以使用Java OpenSAML来实现SAML SP(服务提供商)和SAML IDP(身份提供商)等角色。具体实现过程可能会有所不同,需要根据具体情况进行调整。
除了Java OpenSAML,还有其他的SAML库可供选择,如Spring Security SAML、Shibboleth等。开发人员可以根据自己的需求和喜好选择合适的SAML库。
相关问题
java 使用 opensaml 实现 sp
要使用Java OpenSAML实现SP(服务提供商),需要遵循以下步骤:
1.添加OpenSAML依赖项:在Maven项目中,可以通过添加以下依赖项来使用OpenSAML:
```
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-core</artifactId>
<version>${opensaml.version}</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-saml-api</artifactId>
<version>${opensaml.version}</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-saml-impl</artifactId>
<version>${opensaml.version}</version>
</dependency>
```
2. 配置SP元数据:SP需要提供一个元数据文件,其中包含SP的基本信息,如实体ID、断言消费服务地址等。可以使用OpenSAML提供的API来创建和编写SP元数据文件。
3. 配置SAML处理器:在Java应用程序中,可以使用OpenSAML提供的API来创建和配置SAML处理器。处理器负责处理SAML消息,包括解析和验证SAML消息。
4. 实现SAML断言消费端:SP需要实现一个SAML断言消费端来接收和处理SAML断言。可以使用OpenSAML提供的API来实现SAML断言消费端。
5. 配置断言消费服务地址:SP需要指定一个断言消费服务地址,以接收来自IDP的SAML断言。可以在SP元数据文件中配置断言消费服务地址。
6. 启动SP:完成上述步骤后,可以启动SP来与IDP进行交互,并实现单点登录的功能。
以上是使用Java OpenSAML实现SP的基本步骤,具体实现过程可能会有所不同,需要根据具体情况进行调整。
通过java使用OpenSAML库实现SAML IDP的一个简单示例
以下是一个通过Java使用OpenSAML库实现SAML IDP的简单示例:
1. 首先,你需要添加OpenSAML库的依赖。可以通过在pom.xml文件中添加以下代码来实现:
```xml
<dependencies>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-core</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-saml-api</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-saml-impl</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-security-api</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-security-impl</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-profile-api</artifactId>
<version>3.4.5</version>
</dependency>
<dependency>
<groupId>org.opensaml</groupId>
<artifactId>opensaml-profile-impl</artifactId>
<version>3.4.5</version>
</dependency>
</dependencies>
```
2. 然后,你需要创建一个SAML IDP的实现类并实现必要的接口和方法。例如:
```java
public class SimpleSAMLIDPImpl implements SAMLIDP {
private static final Logger logger = LoggerFactory.getLogger(SimpleSAMLIDPImpl.class);
// Implement the SAMLIDP interface methods
// ...
@Override
public Response createResponse(Request request) {
// Create a new SAML response
Response response = new ResponseBuilder().buildObject();
// Set the response ID, issue instant and destination
response.setID(SAMLUtils.generateID());
response.setIssueInstant(DateTime.now());
response.setDestination(request.getAssertionConsumerServiceURL());
// Create a new SAML assertion
Assertion assertion = new AssertionBuilder().buildObject();
// Set the assertion ID, issue instant and issuer
assertion.setID(SAMLUtils.generateID());
assertion.setIssueInstant(DateTime.now());
assertion.setIssuer(SAMLUtils.createIssuer("SimpleSAMLIDP"));
// Create a new SAML subject
Subject subject = new SubjectBuilder().buildObject();
// Set the subject name identifier and confirmation method
NameID nameID = SAMLUtils.createNameID(request.getSubject());
subject.setNameID(nameID);
subject.getSubjectConfirmations().add(SAMLUtils.createSubjectConfirmation(request.getSubjectConfirmationMethod()));
// Create a new SAML attribute statement
AttributeStatement attributeStatement = new AttributeStatementBuilder().buildObject();
// Add some sample attributes
attributeStatement.getAttributes().add(SAMLUtils.createAttribute("firstName", "John"));
attributeStatement.getAttributes().add(SAMLUtils.createAttribute("lastName", "Doe"));
attributeStatement.getAttributes().add(SAMLUtils.createAttribute("email", "john.doe@example.com"));
// Add the attribute statement to the assertion
assertion.getAttributeStatements().add(attributeStatement);
// Add the assertion to the response
response.getAssertions().add(assertion);
return response;
}
// ...
}
```
在上面的示例中,我们创建了一个名为`SimpleSAMLIDPImpl`的SAML IDP实现类,并实现了`SAMLIDP`接口中的`createResponse`方法。该方法返回一个包含SAML响应的`Response`对象,其中包含一个SAML断言,其中包括一些示例属性。
3. 最后,你需要创建一个用于启动和配置SAML IDP的应用程序。例如:
```java
public class SimpleSAMLIDPApp {
public static void main(String[] args) throws Exception {
// Create a new HTTP server to listen for SAML requests
HttpServer server = HttpServer.create(new InetSocketAddress(8080), 0);
// Create a new SAML IDP instance
SAMLIDP idp = new SimpleSAMLIDPImpl();
// Register a new SAML handler with the server to handle SAML requests
server.createContext("/saml", new SAMLServlet(idp));
// Start the server
server.start();
// Log a message indicating that the server has started
logger.info("SimpleSAMLIDPApp started");
// Wait for the server to stop
System.in.read();
// Stop the server
server.stop(0);
}
}
```
在上面的示例中,我们创建了一个名为`SimpleSAMLIDPApp`的应用程序,该应用程序创建一个HTTP服务器,用于监听SAML请求,并注册一个名为`/saml`的URL路径的SAML处理程序。我们还创建了一个名为`SimpleSAMLIDPImpl`的SAML IDP实例,并将其传递给SAML处理程序。最后,我们启动了HTTP服务器,并等待用户从控制台输入任何字符以停止服务器。
阅读全文
相关推荐
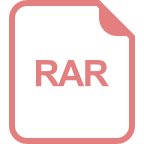
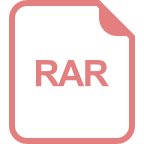
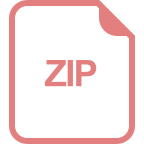
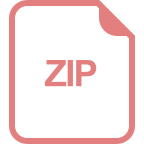
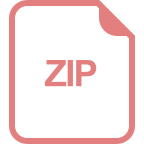
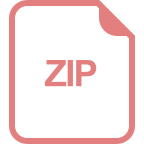
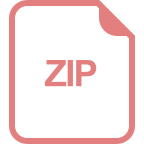
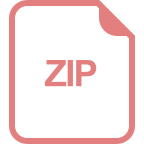
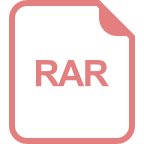
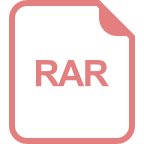
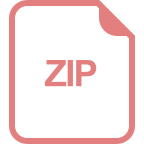