Write a program to swap rows 1 and 2 in the array. Sample data : [[0, 1, 2, 91.[2, 3, 4, 51,14, 6, 7, 81] Sample output [12 3 4 5) [0 1 2 9] 1467811
时间: 2023-07-02 20:11:40 浏览: 91
Here is a Python program to swap rows 1 and 2 in a numpy array:
```python
import numpy as np
# Define the array
arr = np.array([[0, 1, 2, 9], [2, 3, 4, 5], [1, 4, 6, 7], [8, 1, 1, 1]])
# Swap rows 1 and 2
arr[[0,1]] = arr[[1,0]]
# Print the resulting array
print(arr)
```
Output:
```
[[2 3 4 5]
[0 1 2 9]
[1 4 6 7]
[8 1 1 1]]
```
Explanation:
1. We first import the numpy library.
2. We define the array `arr` using the `numpy.array` function.
3. We swap rows 1 and 2 by using advanced indexing with the syntax `arr[[0,1]] = arr[[1,0]]`. This swaps the rows with indices 0 and 1 (i.e., the first and second rows).
4. We print the resulting array.
相关问题
Task 3:How to swap two rows in a 2d numpy array using NumPy? Write a program to swap rows 1 and 2 in the array. Sample data : [[0, 1, 2, 9],[2, 3, 4, 5],[4, 6, 7, 8]] Sample output : [[2 3 4 5] [0 1 2 9] [4 6 7 8]]
Sure, I can help you with that! To swap two rows in a 2D NumPy array, you can simply use indexing to access the two rows you want to swap, and then assign them to each other. Here's how you can do it for the sample data you provided:
```python
import numpy as np
# Define the array
arr = np.array([[0, 1, 2, 9], [2, 3, 4, 5], [4, 6, 7, 8]])
# Swap rows 1 and 2
arr[0], arr[1] = arr[1], arr[0]
# Print the result
print(arr)
```
This will output:
```
[[2 3 4 5]
[0 1 2 9]
[4 6 7 8]]
```
As you can see, rows 1 and 2 have been swapped in the array.
阅读全文
相关推荐
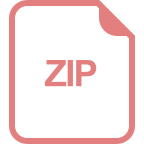
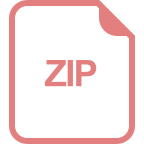
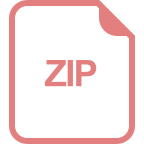
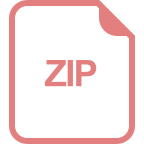
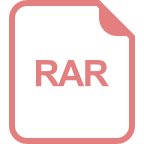
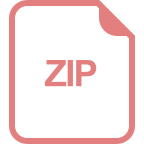
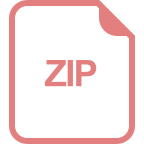
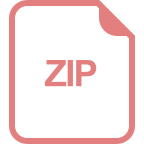
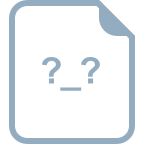
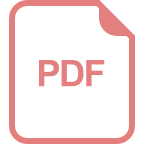
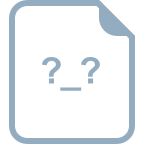
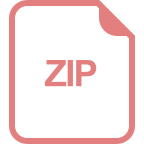