#include<iostream> using namespace std; //Box类的定义及成员函数书写 //***begin*** class Box { private: int length; int width; int heigh; public: Box(); Box(float len,float wid,float hei); double getVolume(); }; Box::Box(float len,float wid,float hei):length(len),width(wid),heigh(hei){} double Box::getVolume() { return heigh*lenth*width; } //***end*** int main(int argc, char *argv[]) { Box b1(4); Box b2(4,5); Box b3(4,10,8); cout<<b1.getVolume()<<","<<b2.getVolume()<<","<<b3.getVolume(); }有什么语法错误
时间: 2024-04-01 07:31:50 浏览: 44
这段代码存在如下语法错误:
1. 在类定义中,Box类的私有成员变量中,lenth应该是length。
2. 在Box类的构造函数Box(float len,float wid,float hei)中,应该在冒号后面使用成员初始化列表来初始化类的数据成员,例如:
```
Box::Box(float len, float wid, float hei) : length(len), width(wid), heigh(hei) {}
```
3. 在Box类的成员函数getVolume()中,应该将lenth改为length,例如:
```
double Box::getVolume() {
return heigh * length * width;
}
```
4. 在主函数中,Box b1(4)的定义中,应该使用三个参数的Box构造函数,例如:
```
Box b1(4, 4, 4);
```
修改后的代码如下:
```
#include<iostream>
using namespace std;
//Box类的定义及成员函数书写
class Box {
private:
int length;
int width;
int heigh;
public:
Box();
Box(float len, float wid, float hei);
double getVolume();
};
// Box类的构造函数的实现
Box::Box(float len, float wid, float hei) : length(len), width(wid), heigh(hei) {}
// Box类的成员函数getVolume()的实现
double Box::getVolume() {
return heigh * length * width;
}
int main(int argc, char *argv[]) {
Box b1(4, 4, 4);
Box b2(4, 5, 4);
Box b3(4, 10, 8);
cout << b1.getVolume() << "," << b2.getVolume() << "," << b3.getVolume();
return 0;
}
```
注意:为了方便起见,这里使用了构造函数中的默认值来简化代码,如果需要使用不同的默认值,可以适当调整构造函数的实现。
相关推荐
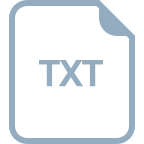
















