python怎么调用prophet模型
时间: 2023-05-19 22:00:57 浏览: 178
你可以使用以下代码调用 Prophet 模型:
```python
from fbprophet import Prophet
# 创建 Prophet 模型
model = Prophet()
# 训练模型
model.fit(train_data)
# 预测未来数据
future = model.make_future_dataframe(periods=365)
forecast = model.predict(future)
# 输出预测结果
print(forecast[['ds', 'yhat', 'yhat_lower', 'yhat_upper']])
```
其中,train_data 是你的训练数据,可以是一个 Pandas DataFrame,包含两列:ds 表示时间,y 表示对应的数值。periods 表示预测未来的时间范围,可以根据需要进行调整。预测结果包含四列:ds 表示时间,yhat 表示预测值,yhat_lower 和 yhat_upper 表示预测值的下限和上限。
相关问题
python prophet模型
Prophet是Facebook开源的一款时间序列预测模型,可以预测趋势、季节性和节假日效应等。它采用了加法模型,将时间序列分解为趋势、季节性和节假日效应三个部分,然后对其进行建模和预测。Prophet还提供了多种可视化工具,方便用户对预测结果进行分析和展示。使用Prophet模型可以很方便地对时间序列数据进行建模和预测,特别适合对季节性强、周期性明显的数据进行预测。
python中prophet模型自动调参到最优代码
Prophet模型的自动调参可以使用`prophet.diagnostics.cross_validation`和`prophet.diagnostics.performance_metrics`两个函数来实现。
以下是一个基本的自动调参代码示例:
```python
from fbprophet import Prophet
from fbprophet.diagnostics import cross_validation, performance_metrics
# 读取数据并处理成Prophet所需的格式
df = pd.read_csv('data.csv')
df = df.rename(columns={'ds': 'ds', 'y': 'y'})
# 定义模型并设置参数范围
model = Prophet(changepoint_prior_scale=0.01, seasonality_prior_scale=10)
params_grid = {'changepoint_prior_scale': [0.001, 0.01, 0.1],
'seasonality_prior_scale': [0.1, 1, 10]}
# 进行交叉验证并计算性能指标
cv_results = cross_validation(model=model, horizon='30 days', period='180 days', initial='730 days')
perf_metrics = performance_metrics(cv_results)
# 找到最优参数组合
best_params = perf_metrics[['changepoint_prior_scale', 'seasonality_prior_scale']].mean().to_dict()
# 使用最优参数训练模型
model = Prophet(**best_params)
model.fit(df)
```
在这个例子中,我们定义了一个Prophet模型并设置了两个参数的范围`changepoint_prior_scale`和`seasonality_prior_scale`。然后,我们使用`cross_validation`函数进行交叉验证,并使用`performance_metrics`函数计算性能指标。最后,我们从性能指标中找到平均最佳参数组合,并使用这些参数训练模型。
需要注意的是,这只是一个基本示例,实际上,还需要对其他参数进行调整,以确保使用的模型能够最好地适应数据。
相关推荐
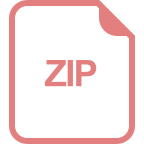
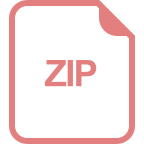












