C#互斥锁
时间: 2023-07-12 12:23:15 浏览: 113
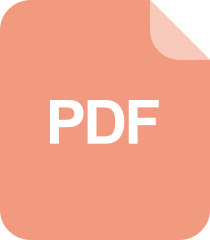
C#多线程中如何运用互斥锁Mutex
C#中的互斥锁是一种同步机制,用于保护共享资源的并发访问。当一个线程拥有互斥锁时,其他线程无法同时拥有该锁,只能等待该线程释放该锁后才能获得。这样可以防止多个线程同时访问共享资源,避免数据竞争和不可预测的行为。
在C#中,可以使用System.Threading命名空间中的Mutex类来创建互斥锁。下面是一个示例代码,演示了如何使用互斥锁来保护共享资源的访问:
```
using System;
using System.Threading;
public class Example
{
private static Mutex mutex = new Mutex(); // 创建互斥锁对象
public static void Main()
{
for (int i = 0; i < 5; i++)
{
Thread myThread = new Thread(new ThreadStart(MyThreadProc));
myThread.Name = String.Format("Thread{0}", i + 1);
myThread.Start();
}
}
private static void MyThreadProc()
{
Console.WriteLine("{0} is waiting for the mutex", Thread.CurrentThread.Name);
mutex.WaitOne(); // 等待互斥锁
Console.WriteLine("{0} has entered the critical section", Thread.CurrentThread.Name);
// 访问共享资源
Console.WriteLine("{0} is leaving the critical section", Thread.CurrentThread.Name);
mutex.ReleaseMutex(); // 释放互斥锁
}
}
```
在上面的示例中,创建了一个互斥锁对象mutex,并在MyThreadProc方法中使用mutex.WaitOne()等待互斥锁,使用mutex.ReleaseMutex()释放互斥锁。这样可以保证只有一个线程可以进入“critical section”,避免了多个线程同时访问共享资源的问题。
阅读全文
相关推荐
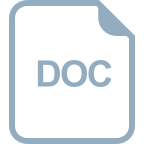
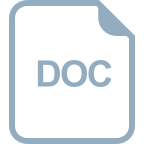
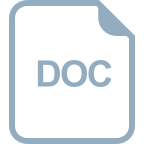
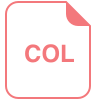



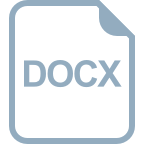
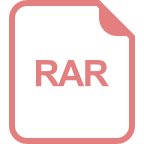
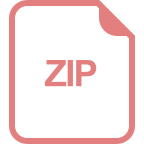
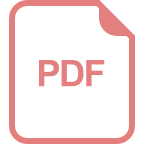
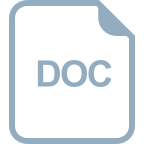


