import random import numpy as np import matplotlib.pyplot as plt 生成随机坐标点 def generate_points(num_points): points = [] for i in range(num_points): x = random.uniform(-10, 10) y = random.uniform(-10, 10) points.append([x, y]) return points 计算欧几里得距离 def euclidean_distance(point1, point2): return np.sqrt(np.sum(np.square(np.array(point1) - np.array(point2)))) K-means算法实现 def kmeans(points, k, num_iterations=100): num_points = len(points) # 随机选择k个点作为初始聚类中心 centroids = random.sample(points, k) # 初始化聚类标签和距离 labels = np.zeros(num_points) distances = np.zeros((num_points, k)) for i in range(num_iterations): # 计算每个点到每个聚类中心的距离 for j in range(num_points): for l in range(k): distances[j][l] = euclidean_distance(points[j], centroids[l]) # 根据距离将点分配到最近的聚类中心 for j in range(num_points): labels[j] = np.argmin(distances[j]) # 更新聚类中心 for l in range(k): centroids[l] = np.mean([points[j] for j in range(num_points) if labels[j] == l], axis=0) return labels, centroids 生成坐标点 points = generate_points(100) 对点进行K-means聚类 k_values = [2, 3, 4] for k in k_values: labels, centroids = kmeans(points, k) # 绘制聚类结果 colors = [‘r’, ‘g’, ‘b’, ‘y’, ‘c’, ‘m’] for i in range(k): plt.scatter([points[j][0] for j in range(len(points)) if labels[j] == i], [points[j][1] for j in range(len(points)) if labels[j] == i], color=colors[i]) plt.scatter([centroid[0] for centroid in centroids], [centroid[1] for centroid in centroids], marker=‘x’, color=‘k’, s=100) plt.title(‘K-means clustering with k={}’.format(k)) plt.show()import numpy as np import pandas as pd import matplotlib.pyplot as plt from sklearn.cluster import KMeans from sklearn.datasets import load_iris 载入数据集 iris = load_iris() X = iris.data y = iris.target K-means聚类 kmeans = KMeans(n_clusters=3, random_state=0).fit(X) 可视化结果 plt.scatter(X[:, 0], X[:, 1], c=kmeans.labels_) plt.xlabel(‘Sepal length’) plt.ylabel(‘Sepal width’) plt.title(‘K-means clustering on iris dataset’) plt.show()从聚类算法的评价指标对结果进行分析
时间: 2024-01-07 10:02:33 浏览: 157
常用的聚类算法评价指标有以下几种:
1. SSE(Sum of Squared Errors):簇内误差平方和,即簇内各点到簇中心的距离平方和,SSE越小表示簇内数据越紧密。
2. Silhouette Coefficient(轮廓系数):用于衡量样本聚类的密集程度,取值范围在[-1,1]之间,越接近1表示聚类效果越好。
3. Calinski-Harabasz Index(方差比率准则):通过计算类间离散度与类内离散度的比值来评估聚类的效果,CHI越大表示聚类效果越好。
4. Davies-Bouldin Index(DBI):通过计算簇内的平均距离和簇间的距离来衡量聚类效果,DBI越小表示聚类效果越好。
对于以上评价指标,可以根据具体的业务场景和需求选择合适的指标进行评估。在实际使用中,可以采用交叉验证等方法来验证聚类算法的效果。
相关问题
我用Cython封装了一个来自segment-anything-master项目里的.pth模型,我的.pyx文件是这么写的import sys import numpy as np import matplotlib import matplotlib.pyplot as plt import cv2 from typing import Any, Dict, List, Optional, Tuple sys.path.append("/app/PythonProjects/segment-anything-main") from segment_anything import sam_model_registry, SamAutomaticMaskGenerator, SamPredictor # model.pyxcdef class SamModel: def __init__(self, str model_type,str sam_checkpoint, str device = "cpu", points_per_side: Optional[int] = 32, points_per_batch: int = 64, point_grids: Optional[List[np.ndarray]] = None): self.sam = sam_model_registry[model_type](checkpoint=sam_checkpoint) self.sam.to(device) self.mask_generator = SamAutomaticMaskGenerator(self.sam,points_per_side = points_per_side, points_per_batch = points_per_batch, point_grids = point_grids) def __getmodel__(self): return self.sam def __callmodel__( self, input: np.array ): masks = self.mask_generator.generate(input) return masks,我的setup.py文件是这样写的:from distutils.core import setup from distutils.extension import Extension import Cython from Cython.Build import cythonize import numpy extensions = [ Extension('models.model', ['/app/PythonProjects/segment-anything-main/SamCython/models/model.pyx'], include_dirs=[numpy.get_include()]), ] setup( name='models', ext_modules=cythonize(extensions), ),给我一篇C++调动这个模型的实例
好的,以下是一个简单的C++调用这个模型的示例代码:
```cpp
#include <Python.h>
#include <numpy/arrayobject.h>
#include <iostream>
int main()
{
// 初始化 Python 解释器
Py_Initialize();
// 加载 NumPy 模块
import_array();
// 加载 Python 模块和函数
PyObject* pModule = PyImport_ImportModule("models.model");
if (!pModule) {
std::cerr << "Failed to import module" << std::endl;
return 1;
}
PyObject* pFunc = PyObject_GetAttrString(pModule, "__callmodel__");
if (!pFunc || !PyCallable_Check(pFunc)) {
std::cerr << "Failed to get function" << std::endl;
return 1;
}
// 准备输入数据
int width = 640;
int height = 480;
npy_intp dims[3] = { height, width, 3 };
unsigned char* inputData = new unsigned char[width * height * 3];
// 填充 inputData 数据
// 创建 NumPy 数组
PyObject* pInputArray = PyArray_SimpleNewFromData(
3, dims, NPY_UINT8, inputData);
// 调用函数
PyObject* pOutputArray = PyObject_CallFunction(pFunc, "O", pInputArray);
if (!pOutputArray) {
std::cerr << "Failed to call function" << std::endl;
return 1;
}
// 获取输出数据
unsigned char* outputData = PyArray_DATA((PyArrayObject*)pOutputArray);
// 处理 outputData 数据
// 释放资源
Py_DECREF(pInputArray);
Py_DECREF(pOutputArray);
Py_DECREF(pFunc);
Py_DECREF(pModule);
Py_Finalize();
delete[] inputData;
return 0;
}
```
需要注意的是,在编译时,需要链接 Python 和 NumPy 库。可以使用以下命令进行编译:
```
g++ -o main main.cpp -I/usr/include/python3.8 -lpython3.8 -lpython3 -lm -ldl -I/usr/local/lib/python3.8/dist-packages/numpy/core/include -L/usr/local/lib/python3.8/config-3.8-x86_64-linux-gnu -lpython3.8 -lcrypt -lpthread -ldl -lutil -lm -Wno-unused-result
```
其中,`-I` 参数指定 Python 和 NumPy 的头文件路径,`-l` 参数指定 Python 的库文件和 NumPy 的库文件(需要根据自己的 Python 版本和安装路径进行调整)。
现有1500个二维空间的数据点,import time as time import numpy as np import matplotlib.pyplot as plt import mpl_toolkits.mplot3d.axes3d as p3 from sklearn.datasets import make_swiss_roll # Generate data (swiss roll dataset) n_samples = 1500 noise = 0.05 X, _ = make_swiss_roll(n_samples, noise=noise) fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.scatter(X[:, 0], X[:, 1], X[:, 2], cmap=plt.cm.Spectral)
这段代码是用来生成一个“瑞士卷”数据集并进行可视化的。数据集中包含1500个点,每个点有3个特征(x、y、z坐标)。这个数据集可以用来测试和比较不同的数据分析和机器学习算法。在这段代码中,使用了sklearn库中的make_swiss_roll函数来生成数据集,并使用matplotlib库中的3D散点图来进行可视化。
阅读全文
相关推荐
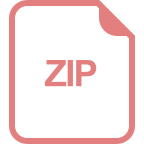
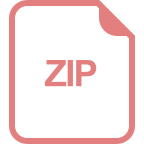
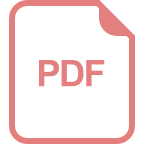












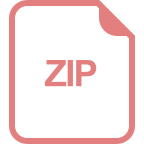