如何将stm32的数据保存到mysql数据库
时间: 2024-05-04 11:16:55 浏览: 33
将STM32的数据保存到MySQL数据库需要以下步骤:
1. 在STM32中配置一个网络连接,使其能够连接到MySQL服务器。可以使用WIFI模块或以太网模块。
2. 在MySQL中创建一个数据库和表,用于存储STM32发送的数据。可以使用MySQL Workbench等工具进行创建。
3. 在STM32中编写代码,将要保存到MySQL数据库中的数据进行格式化和编码。可以使用C语言的MySQL库,如libmysqlclient。
4. 在STM32中通过网络连接将数据传输到MySQL服务器。可以使用MySQL的C API函数进行数据插入操作。
下面是一个基本的示例代码:
```c
#include <mysql/mysql.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define DB_HOST "localhost"
#define DB_USER "root"
#define DB_PASS "password"
#define DB_NAME "mydatabase"
int main()
{
MYSQL *conn;
MYSQL_RES *res;
MYSQL_ROW row;
char *server = DB_HOST;
char *user = DB_USER;
char *password = DB_PASS;
char *database = DB_NAME;
conn = mysql_init(NULL);
if (!mysql_real_connect(conn, server, user, password, database, 0, NULL, 0))
{
fprintf(stderr, "%s\n", mysql_error(conn));
exit(1);
}
if (mysql_query(conn, "CREATE TABLE IF NOT EXISTS data (id INT PRIMARY KEY AUTO_INCREMENT, value INT)"))
{
fprintf(stderr, "%s\n", mysql_error(conn));
exit(1);
}
char query[100];
sprintf(query, "INSERT INTO data (value) VALUES (%d)", 123);
if (mysql_query(conn, query))
{
fprintf(stderr, "%s\n", mysql_error(conn));
exit(1);
}
mysql_close(conn);
exit(0);
}
```
在上面的代码中,我们通过MySQL C API连接到数据库,在数据库中创建一个名为data的表,然后插入一个值为123的记录。
需要注意的是,此代码仅为示例代码,实际应用中需要根据具体需求进行修改和扩展。
相关推荐
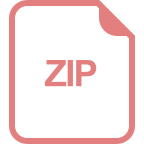
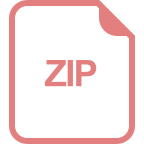














