jsp Servlet上传文件
时间: 2023-07-31 18:08:12 浏览: 99
在JSP中使用Servlet来处理文件上传是一种常见的做法。以下是一个简单的示例代码:
1. 创建一个JSP页面(upload.jsp),用于显示文件上传表单:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件上传</title>
</head>
<body>
<h2>文件上传</h2>
<form action="upload" method="post" enctype="multipart/form-data">
<input type="file" name="file" id="file">
<input type="submit" value="上传">
</form>
</body>
</html>
```
2. 创建一个Servlet(UploadServlet),用于处理文件上传请求:
```java
import java.io.File;
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
@WebServlet("/upload")
@MultipartConfig(fileSizeThreshold = 1024 * 1024 * 2, // 2MB
maxFileSize = 1024 * 1024 * 10, // 10MB
maxRequestSize = 1024 * 1024 * 50) // 50MB
public class UploadServlet extends HttpServlet {
private static final String UPLOAD_DIR = "uploads";
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String applicationPath = request.getServletContext().getRealPath("");
String uploadPath = applicationPath + File.separator + UPLOAD_DIR;
File uploadDir = new File(uploadPath);
if (!uploadDir.exists()) {
uploadDir.mkdir();
}
Part filePart = request.getPart("file");
String fileName = getFileName(filePart);
String filePath = uploadPath + File.separator + fileName;
filePart.write(filePath);
response.getWriter().println("文件上传成功");
}
private String getFileName(Part part) {
String contentDisposition = part.getHeader("content-disposition");
String[] elements = contentDisposition.split(";");
for (String element : elements) {
if (element.trim().startsWith("filename")) {
return element.substring(element.indexOf('=') + 1).trim().replace("\"", "");
}
}
return null;
}
}
```
在上述代码中,使用了`@MultipartConfig`注解来指定文件上传相关的配置,包括文件大小阈值、最大文件大小和最大请求大小。在`doPost`方法中,通过`request.getPart("file")`获取文件的`Part`对象,然后使用`write`方法将文件保存到指定的路径。
3. 部署应用程序到支持Servlet的容器中(如Tomcat),然后访问upload.jsp页面即可看到文件上传表单。
这是一个简单的文件上传示例,你可以根据实际需求进行修改和扩展。同时,请确保在实际开发中处理上传文件时要进行适当的安全验证和错误处理。
阅读全文
相关推荐
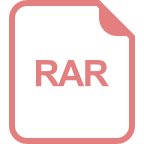
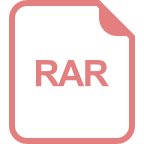
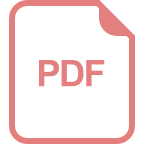
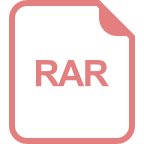
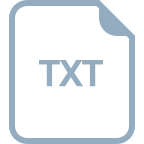
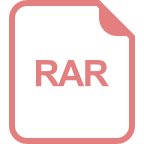
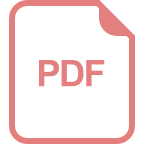
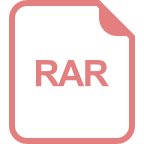
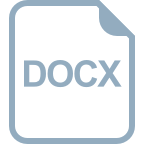
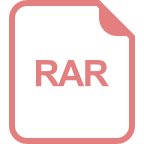
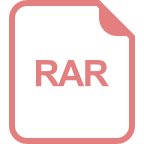
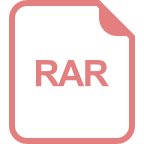
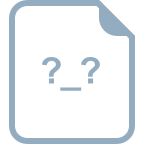
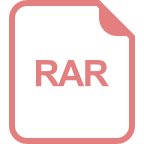