请用c语言编写函数,从键盘输入的英文字母,将其都转换成大写字母,写入到文件中。(以'\n'结尾)
时间: 2024-05-08 12:14:55 浏览: 25
```c
#include <stdio.h>
void writeToFile(char fileName[]) {
char c;
FILE *fp = fopen(fileName, "w");
if (fp == NULL) {
printf("无法打开文件\n");
return;
}
while ((c = getchar()) != '\n') {
if (c >= 'a' && c <= 'z') { // 小写字母转大写字母
c -= 'a' - 'A';
}
fputc(c, fp);
}
fputc('\n', fp); // 写入换行符
fclose(fp);
}
int main() {
char fileName[] = "test.txt";
writeToFile(fileName);
return 0;
}
```
相关问题
c语言:请编写函数,从键盘输入的英文字母,将其都转换成大写字母,写入到文件中。(以'\n'结尾)
好的,这里是一个简单的 C 语言代码实现:
```c
#include <stdio.h>
#include <ctype.h>
void write_uppercase() {
FILE *f = fopen("output.txt", "w");
if (f == NULL) {
printf("Failed to open file.\n");
return;
}
char letter;
while (1) {
printf("请输入一个英文字母:");
scanf(" %c", &letter);
if (!isalpha(letter)) {
break;
}
fputc(toupper(letter), f);
fputc('\n', f);
}
fclose(f);
}
```
这个函数会不断从键盘读入输入,如果输入不是英文字母则停止输入,并将输入的英文字母转换成大写字母写入到文件 `output.txt` 中,并以换行符 `\n` 结尾。需要注意的是,我们在 `scanf` 中加入一个空格,以消耗掉输入缓冲区中的换行符,避免对后续输入造成影响。同样地,可以根据实际需要修改文件名和路径。
C语言编写从键盘输入一个字符串,将其中的小写字母全部转换成大写字母,然后输出到一个磁盘文件“test”中保存。输入的字符串以回车结束。
可以使用C语言中的标准库函数`fgets()`从键盘读入字符串,然后使用循环和条件语句将其中的小写字母转换成大写字母,最后使用文件操作函数将结果写入磁盘文件中。
下面是一个基本的实现示例:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_LENGTH 100
int main() {
char str[MAX_LENGTH];
printf("Enter a string: ");
fgets(str, MAX_LENGTH, stdin); // 从键盘读入字符串
for (int i = 0; str[i] != '\0'; i++) {
if (islower(str[i])) {
str[i] = toupper(str[i]); // 将小写字母转换成大写字母
}
}
FILE *fp = fopen("test", "w"); // 打开文件
if (fp == NULL) {
printf("Failed to open file.\n"); // 打开文件失败
return 1;
}
fputs(str, fp); // 将结果写入文件
fclose(fp); // 关闭文件
printf("Done.\n");
return 0;
}
```
运行程序后,输入一个字符串并按回车,程序将会将其中的小写字母转换成大写字母,并保存到名为“test”的文件中。
相关推荐
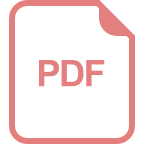
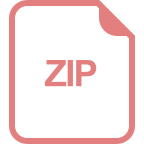
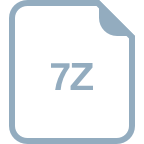












