sdata=sql_data() TypeError: 'module' object is not callable
时间: 2023-10-30 19:38:05 浏览: 136
This error occurs when you try to call a module as a function.
For example, if you have a module named "sql_data" and you try to call it as a function like this:
sdata = sql_data()
Python will raise a TypeError because "sql_data" is not a function, it is a module.
To fix this error, you need to check if you are calling a module or a function. If you want to use a function from the module, you need to import it first and then call the function using the module name and function name separated by a dot.
For example:
from sql_data import my_function
sdata = my_function()
This will import the "my_function" function from the "sql_data" module and call it.
相关问题
这两个Verilog代码可以放在一个.v文件中吗:1.`timescale 1ns / 1ps module Top(clk,sw,led,flag, ADC_sdata, ADC_sclk,ADC_csn,slec_wei,slec_duan); input clk; input [3:0]sw; output reg [7:0] led; input flag; input ADC_sdata; output ADC_sclk,ADC_csn; output [7:0] slec_wei; output [7:0] slec_duan; wire [11:0] adc_res; wire adc_valid; wire [19:0]cout; always@(posedge clk)if(adc_valid) led<=adc_res[11:4]; PmodAD1 U0( .clk(clk), .rst(1’b0), .ADC_sdata(ADC_sdata), .ADC_sclk(ADC_sclk), .ADC_csn(ADC_csn), .adc_res(adc_res), .adc_valid(adc_valid) ); data_ad_pro U1( .sys_clk(clk), .rst_n(1’b1), .pre_data(adc_res[11:4]), .cout(cout) ); display U2( .sys_clk(clk), .rst_n(1’b1), .cout(cout), .sw(sw), .flag(flag), .slec_wei(slec_wei), .slec_duan(slec_duan) ); endmodule ———————2.module PmodAD1( clk,rst, ADC_sdata,ADC_sclk,ADC_csn,adc_res,adc_valid); input clk,rst, ADC_sdata; output reg ADC_sclk,ADC_csn; output reg [11:0] adc_res; output reg adc_valid; reg [7:0] cntr; always@(posedge clk) if(rst)cntr<=0;else if(cntr==34)cntr<=0;else cntr<=cntr+1; always@(posedge clk) case (cntr) 0: ADC_csn<=0; 33: ADC_csn<=1; endcase always@(posedge clk) case(cntr) 34,0,2,4,6,8,10,12,14,16,18,20,22,24,26,28,30,32,33:ADC_sclk<=1; default ADC_sclk<=0; endcase always@(posedge clk) case(cntr) 8: adc_res[11]<= ADC_sdata; 10:adc_res[10]<= ADC_sdata; 12:adc_res[9]<= ADC_sdata; 14:adc_res[8]<= ADC_sdata; 16:adc_res[7]<= ADC_sdata; 18:adc_res[6]<= ADC_sdata; 20:adc_res[5]<= ADC_sdata; 22:adc_res[4]<= ADC_sdata; 24:adc_res[3]<= ADC_sdata; 26:adc_res[2]<= ADC_sdata; 28:adc_res[1]<= ADC_sdata; 30:adc_res[0]<= ADC_sdata; endcase always@(posedge clk)adc_valid<=cntr==32; endmodule
可以将两个模块放在同一个文件中,但需要注意的是,需要在两个模块之间添加一个模块分割符`//----`,否则会报错。同时,建议将两个模块中的端口名进行修改,以免出现冲突。下面是修改后的代码示例:
```
//----Top module----
`timescale 1ns / 1ps
module Top(
input clk,
input [3:0] sw,
output reg [7:0] led,
input flag,
input ADC_sdata,
output ADC_sclk,
output ADC_csn,
output [7:0] slec_wei,
output [7:0] slec_duan
);
wire [11:0] adc_res;
wire adc_valid;
wire [19:0] cout;
always@(posedge clk)
if(adc_valid)
led <= adc_res[11:4];
PmodAD1 U0(
.clk(clk),
.rst(1'b0),
.ADC_sdata(ADC_sdata),
.ADC_sclk(ADC_sclk),
.ADC_csn(ADC_csn),
.adc_res(adc_res),
.adc_valid(adc_valid)
);
data_ad_pro U1(
.sys_clk(clk),
.rst_n(1'b1),
.pre_data(adc_res[11:4]),
.cout(cout)
);
display U2(
.sys_clk(clk),
.rst_n(1'b1),
.cout(cout),
.sw(sw),
.flag(flag),
.slec_wei(slec_wei),
.slec_duan(slec_duan)
);
endmodule
//----PmodAD1 module----
module PmodAD1(
input clk,
input rst,
input ADC_sdata,
output reg ADC_sclk,
output reg ADC_csn,
output reg [11:0] adc_res,
output reg adc_valid
);
reg [7:0] cntr;
always@(posedge clk)
if(rst)
cntr <= 0;
else if(cntr == 34)
cntr <= 0;
else
cntr <= cntr + 1;
always@(posedge clk)
case(cntr)
0: ADC_csn <= 0;
33: ADC_csn <= 1;
endcase
always@(posedge clk)
case(cntr)
34, 0, 2, 4, 6, 8, 10, 12, 14, 16, 18, 20, 22, 24, 26, 28, 30, 32, 33: ADC_sclk <= 1;
default ADC_sclk <= 0;
endcase
always@(posedge clk)
case(cntr)
8: adc_res[11] <= ADC_sdata;
10: adc_res[10] <= ADC_sdata;
12: adc_res[9] <= ADC_sdata;
14: adc_res[8] <= ADC_sdata;
16: adc_res[7] <= ADC_sdata;
18: adc_res[6] <= ADC_sdata;
20: adc_res[5] <= ADC_sdata;
22: adc_res[4] <= ADC_sdata;
24: adc_res[3] <= ADC_sdata;
26: adc_res[2] <= ADC_sdata;
28: adc_res[1] <= ADC_sdata;
30: adc_res[0] <= ADC_sdata;
endcase
always@(posedge clk)
adc_valid <= cntr == 32;
endmodule
```
int generate_frag_data(void){ #if !defined JF return 0; #else int index=0; int data_len=PDU_FRAG_DATA_LEN; memset(frag_data_buf,0,sizeof(frag_data_buf)); MN_printf(0, "generate_frag_data frag_num=%d\r\n",frag_num); #ifdef FIRST_FRAG_ADD_EXTRA_DATA if(FRAG_NUM_START==frag_num){ uint8_t max_min_value[2]; get_sample_data_max_min_value(max_min_value); float v_min=computeMvScale_f(max_min_value[1]); float v_max=1600;//computeMvScale_f(max_min_value[0]); uint8_t * v_max_fp=(uint8_t *)&v_max; uint8_t * v_min_fp=(uint8_t *)&v_min; index=first_frag_add_extra_data((uint8_t *)frag_data_buf,v_min_fp,v_max_fp); data_len+=FIRST_FRAG_EXTRA_DATA_LEN; } #endif int frag_src_data_num= MAX_SAMP_DATA_LEN * MAX_SAMP_BUF_NUM / FRAG_TOTAL_NUM; for(int i=0;i<frag_src_data_num;i++){ int frag_src_data_index= frag_src_data_num*(frag_num-1)+i; int sdata_item_index= frag_src_data_index/MAX_SAMP_DATA_LEN; int sdata_index=frag_src_data_index % MAX_SAMP_DATA_LEN; uint8_t data=sample_jufang_buf.sdata_item[sdata_item_index].sdata[sdata_index]; float data_f=computeMvScale_f(data); memcpy(&frag_data_buf[index+i*4],(uint8_t *)&data_f,4); /*if(i%250==0){ MN_printf(0, "generate_frag_data i=%d\r\n",i); MN_printf(0, "generate_frag_data frag_src_data_num=%d\r\n",frag_src_data_num); MN_printf(0, "generate_frag_data frag_src_data_index=%d\r\n",frag_src_data_index); MN_printf(0, "generate_frag_data sdata_item_index=%d\r\n",sdata_item_index); MN_printf(0, "generate_frag_data sdata_index=%d\r\n",sdata_index); MN_printf(0, "generate_frag_data index+i*4=%d\r\n",index+i*4); MN_printf(0, "generate_frag_data data=%2x\r\n",data); MN_printf(0, "generate_frag_data frag_data_buf[index+i*4]=%2x\r\n",frag_data_buf[index+i*4]); MN_printf(0, "generate_frag_data frag_data_buf[index+i*4+1]=%2x\r\n",frag_data_buf[index+i*4+1]); MN_printf(0, "generate_frag_data frag_data_buf[index+i*4+2]=%2x\r\n",frag_data_buf[index+i*4+2]); MN_printf(0, "generate_frag_data frag_data_buf[index+i*4+3]=%2x\r\n",frag_data_buf[index+i*4+3]); #if defined SAMPLE_DATA_FLOAT_VALUE_UPLOAD float fReceive; *((char *)(&fReceive)) = frag_data_buf[index+i*4]; *((char *)(&fReceive) + 1) = frag_data_buf[index+i*4+1]; *((char *)(&fReceive) + 2) = frag_data_buf[index+i*4+2]; *((char *)(&fReceive) + 3) = frag_data_buf[index+i*4+3]; uint32_t a = (uint32_t)(fReceive*1000); MN_printf(0, "sample_data_float_value=%ld\r\n",a); #endif }*/ } return data_len; #endif }
这段代码是用于生成分段数据的,它会根据不同的条件生成不同的数据。其中,宏定义 JF 的值决定了函数的返回值,如果 JF 未定义,则返回 0,否则执行后续操作。变量 frag_num 表示分段数据的编号,变量 frag_src_data_num 表示每个分段数据包含的原始数据个数。函数先清空了一个名为 frag_data_buf 的缓冲区,然后逐个读取原始数据,将其转换为浮点数并存储到缓冲区中,最终返回生成的数据长度。其中,还有一些条件编译的代码块,用于在特定情况下添加额外的数据。
阅读全文
相关推荐
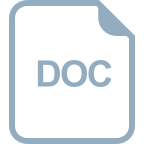
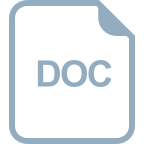
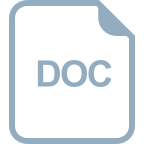


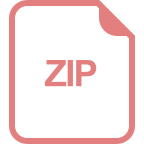










