C#数据库项目实例
时间: 2023-07-12 13:08:22 浏览: 60
以下是一个简单的C#数据库项目实例:
1.创建一个Windows窗体应用程序
2.在Visual Studio中打开“工具”窗口,选择“NuGet程序包管理器”,搜索并安装“Microsoft.Data.SqlClient”包。
3.在窗体上添加一个DataGridView和一些文本框和按钮。
4.创建一个数据库,并创建一个表,例如“学生”。表包含“ID”、“姓名”和“年龄”列。
5.在C#代码中,使用SqlConnection连接到数据库,使用SqlDataAdapter填充DataGridView,使用SqlCommand插入、更新和删除数据。
6.在窗体的Load事件中,使用SqlDataAdapter从数据库中检索数据,并使用DataGridView显示在窗体上。
7.在“添加”按钮的Click事件中,使用SqlCommand将文本框中的数据插入到数据库中,并使用SqlDataAdapter更新DataGridView。
8.在“更新”按钮的Click事件中,使用SqlCommand更新数据库中的数据,并使用SqlDataAdapter更新DataGridView。
9.在“删除”按钮的Click事件中,使用SqlCommand删除数据库中的数据,并使用SqlDataAdapter更新DataGridView。
下面是示例代码:
```
using System;
using System.Data;
using System.Data.SqlClient;
using System.Windows.Forms;
namespace DatabaseProject
{
public partial class Form1 : Form
{
private string connectionString = "Data Source=YourServer;Initial Catalog=YourDatabase;Integrated Security=True";
private SqlConnection connection;
private SqlDataAdapter adapter;
private DataTable table;
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
connection = new SqlConnection(connectionString);
adapter = new SqlDataAdapter("SELECT * FROM 学生", connection);
table = new DataTable();
adapter.Fill(table);
dataGridView1.DataSource = table;
}
private void buttonAdd_Click(object sender, EventArgs e)
{
string name = textBoxName.Text;
int age = int.Parse(textBoxAge.Text);
SqlCommand command = new SqlCommand("INSERT INTO 学生 (姓名, 年龄) VALUES (@Name, @Age)", connection);
command.Parameters.AddWithValue("@Name", name);
command.Parameters.AddWithValue("@Age", age);
connection.Open();
command.ExecuteNonQuery();
connection.Close();
adapter.Fill(table);
}
private void buttonUpdate_Click(object sender, EventArgs e)
{
int id = int.Parse(textBoxID.Text);
string name = textBoxName.Text;
int age = int.Parse(textBoxAge.Text);
SqlCommand command = new SqlCommand("UPDATE 学生 SET 姓名=@Name, 年龄=@Age WHERE ID=@ID", connection);
command.Parameters.AddWithValue("@Name", name);
command.Parameters.AddWithValue("@Age", age);
command.Parameters.AddWithValue("@ID", id);
connection.Open();
command.ExecuteNonQuery();
connection.Close();
adapter.Fill(table);
}
private void buttonDelete_Click(object sender, EventArgs e)
{
int id = int.Parse(textBoxID.Text);
SqlCommand command = new SqlCommand("DELETE FROM 学生 WHERE ID=@ID", connection);
command.Parameters.AddWithValue("@ID", id);
connection.Open();
command.ExecuteNonQuery();
connection.Close();
adapter.Fill(table);
}
}
}
```
当用户单击“添加”、“更新”或“删除”按钮时,该应用程序将向数据库发出相应的SQL命令,并使用SqlDataAdapter更新DataGridView。
相关推荐
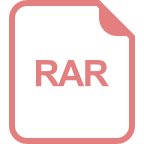
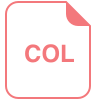
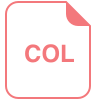
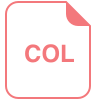
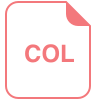
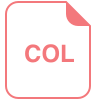









