编写STL程序,读取文件中的候选人名单,随机抽取三位候选人作为获奖者,将获奖人信息输出到屏幕上同时保存到其他文件中
时间: 2024-03-13 15:43:55 浏览: 155
好的,我可以为您提供一个基于STL的C++程序,实现读取文件中的候选人名单,随机抽取三位候选人作为获奖者,将获奖人信息输出到屏幕上同时保存到其他文件中。
以下是程序代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <algorithm>
using namespace std;
int main() {
vector<string> candidates;
string filename = "candidates.txt";
// 读取文件中的候选人名单
ifstream infile(filename);
if (!infile.is_open()) {
cout << "无法打开文件 " << filename << endl;
return 1;
}
string candidate;
while (getline(infile, candidate)) {
candidates.push_back(candidate);
}
infile.close();
// 随机抽取三位候选人作为获奖者
random_shuffle(candidates.begin(), candidates.end());
vector<string> winners(candidates.begin(), candidates.begin() + 3);
// 将获奖人信息输出到屏幕上
cout << "恭喜以下三位候选人获奖:" << endl;
for (const auto& winner : winners) {
cout << winner << endl;
}
// 将获奖人信息保存到其他文件中
string output_filename = "winners.txt";
ofstream outfile(output_filename);
if (!outfile.is_open()) {
cout << "无法打开文件 " << output_filename << endl;
return 1;
}
for (const auto& winner : winners) {
outfile << winner << endl;
}
outfile.close();
return 0;
}
```
以上程序首先通过一个 vector 存储候选人名单。然后,使用 ifstream 从文件中读取候选人名单,并将每个候选人添加到 vector 中。接下来,使用 random_shuffle 随机打乱 vector 中的候选人,然后从 vector 中选出前三位候选人作为获奖者。最后,使用 ofstream 将获奖人信息存储到文件中,并输出到屏幕上。
请注意,程序中的文件名和路径可能需要根据实际情况进行修改。
阅读全文
相关推荐
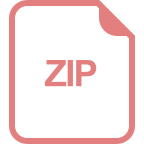
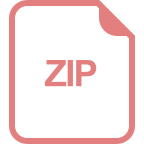
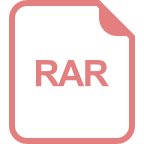
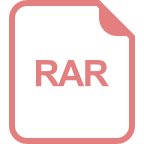
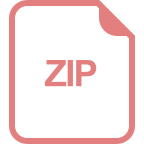
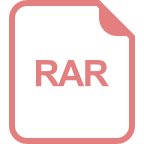
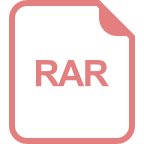
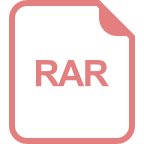
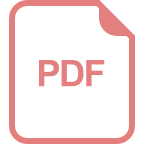
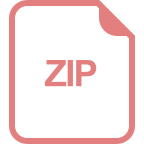
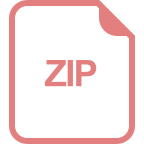