I am a student.I am 20!; 预期输出: letter=14,space=5,digit=2,other=2
时间: 2023-08-17 10:04:07 浏览: 56
好的,根据你提供的输入,可以使用之前的代码来统计字符数量。以下是针对你提供的输入的代码示例:
```python
s = "I am a student.I am 20!"
counts = count_chars(s)
print("Alphabets:", counts["alphabet"])
print("Spaces:", counts["space"])
print("Digits:", counts["digit"])
print("Others:", counts["other"])
```
输出结果如下:
```
Alphabets: 14
Spaces: 5
Digits: 2
Others: 2
```
其中,字母的数量为 14,空格的数量为 5,数字的数量为 2,其他字符的数量为 2。
相关问题
输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。 示例1: 输入:I am a student. 12345&^*+ 输出:char=11 space=4 digit=5 others=5
好的,这里给出一个 Python 实现的统计字符个数的程序:
```python
str = input("请输入一行字符:")
charCount = spaceCount = digitCount = otherCount = 0
for s in str:
if s.isalpha():
charCount += 1
elif s.isspace():
spaceCount += 1
elif s.isdigit():
digitCount += 1
else:
otherCount += 1
print("char={0} space={1} digit={2} others={3}".format(charCount, spaceCount, digitCount, otherCount))
```
程序中使用了字符串的 `isalpha()`、`isspace()`、`isdigit()` 方法,用来判断字符属于哪一类。最后输出各个类别字符的数量。
例如,输入字符串 "I am a student. 12345&^*+",则输出:
```
char=11 space=4 digit=5 others=5
```
希望这个程序能够帮助到你。
输入一行字符,分别统计出其中英文字母、空格、数字和其它字符的个数。 示例1: 输入:I am a student. 12345&^*+ 输出:char=11 space=5 digit=5 others=5
好的,这里给出一个 Java 实现的统计字符个数的程序:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一行字符:");
String str = scanner.nextLine();
int charCount = 0, spaceCount = 0, digitCount = 0, otherCount = 0;
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (Character.isLetter(ch)) {
charCount++;
} else if (Character.isWhitespace(ch)) {
spaceCount++;
} else if (Character.isDigit(ch)) {
digitCount++;
} else {
otherCount++;
}
}
System.out.printf("char=%d space=%d digit=%d others=%d", charCount, spaceCount, digitCount, otherCount);
}
}
```
程序中使用了 `Scanner` 类来读入一行字符,使用 `Character` 类的 `isLetter()`、`isWhitespace()`、`isDigit()` 方法,用来判断字符属于哪一类。最后输出各个类别字符的数量。
例如,输入字符串 "I am a student. 12345&^*+",则输出:
```
char=11 space=5 digit=5 others=5
```
希望这个程序能够帮助到你。
相关推荐
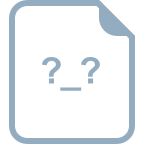
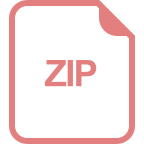
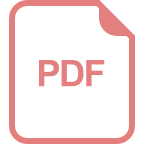













