C# h264h265视频推送
时间: 2023-07-12 15:04:14 浏览: 59
推送H.264/H.265视频的方法,通常需要使用专业的视频编码库,例如FFmpeg或GStreamer。这些库提供了一组API,可以用C#等编程语言访问它们。
具体来说,你需要使用以下步骤:
1. 安装和配置FFmpeg或GStreamer库。
2. 通过C#编写代码,连接到视频源(例如网络摄像头或文件)并打开视频流。
3. 设置编码器参数,例如视频编码方式(H.264/H.265)、分辨率、帧率等。
4. 将视频帧发送到编码器进行编码。
5. 将编码后的视频数据发送到目标位置,例如网络服务器或其他客户端。
这是一个高度技术性的问题,需要一定的编程和视频处理知识。如果你没有相关的经验,可能需要先学习一些相关的知识,并参考一些现有的代码库或示例来实现你的目标。
相关问题
C# H264视频推送
您可以使用 C# 中的FFmpeg库来实现H264视频推送。以下是基本的代码示例:
```csharp
using System;
using System.Diagnostics;
using System.IO;
namespace H264VideoPusher
{
class Program
{
static void Main(string[] args)
{
string ffmpegPath = "path/to/ffmpeg.exe";
string inputVideoPath = "path/to/input.h264";
string outputUrl = "rtmp://your.streaming.server/live/stream_key";
Process ffmpegProcess = new Process();
ffmpegProcess.StartInfo.FileName = ffmpegPath;
ffmpegProcess.StartInfo.Arguments = $"-re -i {inputVideoPath} -c:v copy -f flv {outputUrl}";
ffmpegProcess.StartInfo.RedirectStandardOutput = true;
ffmpegProcess.StartInfo.UseShellExecute = false;
ffmpegProcess.StartInfo.CreateNoWindow = true;
Console.WriteLine($"Starting video push to {outputUrl}...");
ffmpegProcess.Start();
while (!ffmpegProcess.StandardOutput.EndOfStream)
{
Console.WriteLine(ffmpegProcess.StandardOutput.ReadLine());
}
}
}
}
```
这个示例中,我们使用了FFmpeg库来读取H264格式的视频文件,并将其推送到指定的RTMP服务器。要使用这个示例,您需要将`ffmpegPath`变量设置为FFmpeg库的路径,将`inputVideoPath`变量设置为您要推送的H264视频文件的路径,并将`outputUrl`变量设置为您的RTMP服务器URL和流密钥。
请注意,这个示例需要您的机器上安装了FFmpeg库。您可以从FFmpeg官网下载并安装FFmpeg库。
C# h264视频推送类
您可以使用 C# 中的 FFmpeg 库来实现 H264 视频推送。FFmpeg 是一个跨平台的多媒体解决方案,可以实现音视频的编解码、转码、播放等功能。
以下是使用 FFmpeg 实现 H264 视频推送的示例代码:
```csharp
using System;
using System.Diagnostics;
using System.Runtime.InteropServices;
using FFmpeg.AutoGen;
namespace H264Pusher
{
class Program
{
static void Main(string[] args)
{
// 初始化 FFmpeg 库
ffmpeg.av_register_all();
ffmpeg.avformat_network_init();
// 创建输出流的 AVFormatContext 对象
AVFormatContext* outputContext = null;
string outputUrl = "rtmp://example.com/live/stream";
int ret = ffmpeg.avformat_alloc_output_context2(&outputContext, null, "flv", outputUrl);
if (ret < 0)
{
Console.WriteLine("Failed to allocate output context: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 添加视频流
AVStream* videoStream = ffmpeg.avformat_new_stream(outputContext, null);
if (videoStream == null)
{
Console.WriteLine("Failed to create video stream");
return;
}
// 设置视频流参数
AVCodecParameters* codecParams = videoStream->codecpar;
codecParams->codec_id = AVCodecID.AV_CODEC_ID_H264;
codecParams->codec_type = AVMediaType.AVMEDIA_TYPE_VIDEO;
codecParams->width = 1920; // 视频宽度
codecParams->height = 1080; // 视频高度
codecParams->format = (int)AVPixelFormat.AV_PIX_FMT_YUV420P; // 像素格式
codecParams->bit_rate = 2000000; // 码率
codecParams->profile = FF_PROFILE_H264_HIGH; // H264 Profile
codecParams->level = 41; // H264 Level
// 初始化视频编码器
AVCodec* codec = ffmpeg.avcodec_find_encoder(codecParams->codec_id);
if (codec == null)
{
Console.WriteLine("Failed to find codec");
return;
}
AVCodecContext* codecContext = ffmpeg.avcodec_alloc_context3(codec);
if (codecContext == null)
{
Console.WriteLine("Failed to allocate codec context");
return;
}
ret = ffmpeg.avcodec_parameters_to_context(codecContext, codecParams);
if (ret < 0)
{
Console.WriteLine("Failed to copy codec parameters to context: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
ret = ffmpeg.avcodec_open2(codecContext, codec, null);
if (ret < 0)
{
Console.WriteLine("Failed to open codec: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 打开输出流
ret = ffmpeg.avio_open2(&outputContext->pb, outputUrl, AVIO_FLAG_WRITE, null, null);
if (ret < 0)
{
Console.WriteLine("Failed to open output stream: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 写入文件头
ret = ffmpeg.avformat_write_header(outputContext, null);
if (ret < 0)
{
Console.WriteLine("Failed to write output header: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 将视频帧编码并写入输出流
AVFrame* frame = ffmpeg.av_frame_alloc();
byte[] yuvData = new byte[codecParams->width * codecParams->height * 3 / 2]; // YUV数据
int frameCount = 0;
Stopwatch stopwatch = Stopwatch.StartNew();
while (stopwatch.ElapsedMilliseconds < 30000) // 推流30秒钟
{
// 生成一帧随机的 YUV 数据
for (int i = 0; i < yuvData.Length; i++)
{
yuvData[i] = (byte)(i + frameCount);
}
// 将 YUV 数据填充到 AVFrame 中
ffmpeg.av_image_fill_arrays(frame->data, frame->linesize, yuvData, codecParams->format, codecParams->width, codecParams->height, 1);
// 将 AVFrame 编码为 H264 格式
AVPacket packet;
ffmpeg.av_init_packet(&packet);
packet.data = null;
packet.size = 0;
ret = ffmpeg.avcodec_send_frame(codecContext, frame);
if (ret < 0)
{
Console.WriteLine("Failed to send frame to codec: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
while (ret >= 0)
{
ret = ffmpeg.avcodec_receive_packet(codecContext, &packet);
if (ret == AVERROR.AVERROR_EAGAIN || ret == AVERROR.AVERROR_EOF)
{
break;
}
else if (ret < 0)
{
Console.WriteLine("Failed to receive packet from codec: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 将编码后的数据写入输出流
ret = ffmpeg.av_write_frame(outputContext, &packet);
if (ret < 0)
{
Console.WriteLine("Failed to write packet to output stream: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
ffmpeg.av_packet_unref(&packet);
}
frameCount++;
}
// 写入文件尾
ret = ffmpeg.av_write_trailer(outputContext);
if (ret < 0)
{
Console.WriteLine("Failed to write output trailer: " + Marshal.PtrToStringAnsi(ffmpeg.av_err2str(ret)));
return;
}
// 释放资源
ffmpeg.avformat_free_context(outputContext);
ffmpeg.avcodec_free_context(&codecContext);
ffmpeg.av_frame_free(&frame);
}
}
}
```
以上代码仅供参考,具体实现需要根据您的实际需求进行调整。
相关推荐
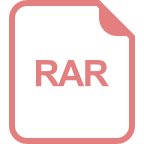
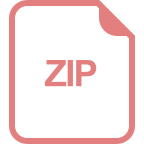
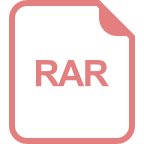












