linux 如何禁止修改文件内容 用C语言代码实现
时间: 2023-12-15 18:05:51 浏览: 39
要用 C 语言代码实现禁止修改 Linux 文件内容的功能,可以使用以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
// Set the file path and content that you want to protect
char* file_path = "/etc/hosts";
char* file_content = "127.0.0.1 localhost\n127.0.1.1 myhostname\n";
// Open the file in read-only mode
FILE* fp = fopen(file_path, "r");
if (fp == NULL) {
printf("Failed to open the file!\n");
return 1;
}
// Read the file content into a buffer
fseek(fp, 0L, SEEK_END);
long file_size = ftell(fp);
rewind(fp);
char* buffer = (char*) malloc(file_size + 1);
fread(buffer, file_size, 1, fp);
buffer[file_size] = '\0';
// Compare the file content with the protected content
if (strcmp(buffer, file_content) != 0) {
printf("The file has been modified! Restoring the original content...\n");
// Open the file in write mode and overwrite the content with the protected content
fp = fopen(file_path, "w");
if (fp == NULL) {
printf("Failed to open the file in write mode!\n");
return 1;
}
fputs(file_content, fp);
fclose(fp);
printf("The file has been restored to its original content!\n");
} else {
printf("The file has not been modified.\n");
}
// Free the buffer and close the file
free(buffer);
fclose(fp);
return 0;
}
```
这段代码首先指定要保护的文件路径和内容,然后打开该文件并读取其内容到一个缓冲区中。接下来,将缓冲区的内容与要保护的内容进行比较,如果不同,则将文件内容恢复为要保护的内容。最后,释放缓冲区并关闭文件。
需要注意的是,这段代码只能保护指定的文件,如果要保护多个文件,需要对代码进行相应的修改。此外,该代码需要以 root 用户身份运行,否则可能无法读写受保护的文件。
相关推荐
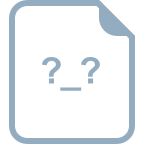
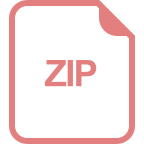














