用C和C++写一段创建多条链表的代码
时间: 2024-05-04 16:18:11 浏览: 74
下面是用 C 语言实现创建多条链表的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node *next;
};
void insert(struct node **head, int data) {
struct node *new_node = (struct node*)malloc(sizeof(struct node));
new_node->data = data;
new_node->next = NULL;
if (*head == NULL) {
*head = new_node;
} else {
struct node *curr = *head;
while (curr->next != NULL) {
curr = curr->next;
}
curr->next = new_node;
}
}
void display(struct node *head) {
printf("List: ");
while (head != NULL) {
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
int main() {
struct node *head1 = NULL;
insert(&head1, 1);
insert(&head1, 2);
insert(&head1, 3);
display(head1);
struct node *head2 = NULL;
insert(&head2, 4);
insert(&head2, 5);
insert(&head2, 6);
display(head2);
return 0;
}
```
上述代码中定义了一个 `struct node` 结构体表示链表节点,其中包含一个整型数据域 `data` 和一个指向下一个节点的指针 `next`。`insert()` 函数用于在链表尾部插入新节点,`display()` 函数用于遍历并打印链表中的所有元素。
在 `main()` 函数中,我们分别创建了两条链表 `head1` 和 `head2`,并对它们进行了插入和打印操作。
阅读全文
相关推荐
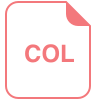
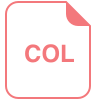
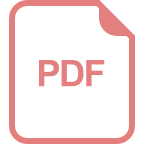
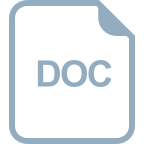
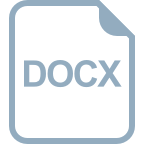
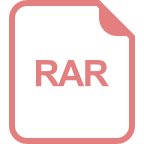
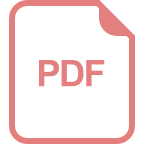
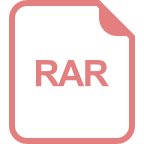
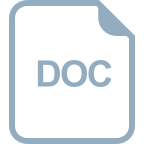
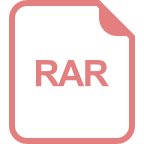
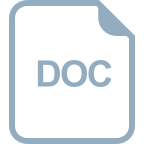
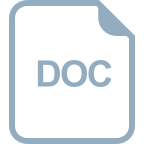
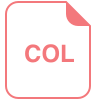
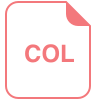
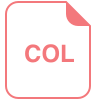
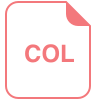