esp32 webrtc 推流 出个demo
时间: 2023-08-24 11:09:50 浏览: 358
好的,这里是一个ESP32 WebRTC推流的Demo:
1. 首先,你需要先在ESP32上安装WebRTC的库。可以使用ESP-IDF的组件来安装,具体方法可以参考官方文档。
2. 接下来,你需要准备一个WebRTC服务器,可以使用开源的Janus Gateway或者Kurento Media Server等。
3. 在ESP32上编写代码,连接到WebRTC服务器并进行推流。下面是一个简单的示例:
```c
#include "esp_camera.h"
#include "esp_log.h"
#include "esp_http_client.h"
#include "esp_websocket_client.h"
#include "esp_timer.h"
#include "esp_wifi.h"
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "driver/gpio.h"
#include "nvs_flash.h"
#include "sdkconfig.h"
#define CAMERA_MODEL_AI_THINKER
#include "camera_pins.h"
#define EXAMPLE_ESP_WIFI_SSID "your_wifi_ssid"
#define EXAMPLE_ESP_WIFI_PASS "your_wifi_password"
#define EXAMPLE_ESP_MAXIMUM_RETRY 10
static const char *TAG = "webrtc_demo";
static esp_http_client_handle_t client;
static esp_websocket_client_handle_t websocket;
static EventGroupHandle_t wifi_event_group;
const int CONNECTED_BIT = BIT0;
static int wifi_retry_num = 0;
static void wifi_event_handler(void* arg, esp_event_base_t event_base, int32_t event_id, void* event_data) {
if (event_base == WIFI_EVENT && event_id == WIFI_EVENT_STA_START) {
esp_wifi_connect();
} else if (event_base == WIFI_EVENT && event_id == WIFI_EVENT_STA_DISCONNECTED) {
if (wifi_retry_num < EXAMPLE_ESP_MAXIMUM_RETRY) {
esp_wifi_connect();
wifi_retry_num++;
ESP_LOGI(TAG, "retry to connect to the AP");
} else {
xEventGroupSetBits(wifi_event_group, CONNECTED_BIT);
}
ESP_LOGI(TAG,"connect to the AP fail");
} else if (event_base == IP_EVENT && event_id == IP_EVENT_STA_GOT_IP) {
ip_event_got_ip_t* event = (ip_event_got_ip_t*) event_data;
ESP_LOGI(TAG, "got ip:%s", ip4addr_ntoa(&event->ip_info.ip));
wifi_retry_num = 0;
xEventGroupSetBits(wifi_event_group, CONNECTED_BIT);
}
}
static void wifi_init_sta(void) {
wifi_event_group = xEventGroupCreate();
tcpip_adapter_init();
ESP_ERROR_CHECK(esp_event_loop_create_default());
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK(esp_wifi_init(&cfg));
ESP_ERROR_CHECK(esp_event_handler_register(WIFI_EVENT, ESP_EVENT_ANY_ID, &wifi_event_handler, NULL));
ESP_ERROR_CHECK(esp_event_handler_register(IP_EVENT, IP_EVENT_STA_GOT_IP, &wifi_event_handler, NULL));
wifi_config_t wifi_config = {
.sta = {
.ssid = EXAMPLE_ESP_WIFI_SSID,
.password = EXAMPLE_ESP_WIFI_PASS,
},
};
ESP_LOGI(TAG, "Connecting to %s", wifi_config.sta.ssid);
ESP_ERROR_CHECK(esp_wifi_set_mode(WIFI_MODE_STA));
ESP_ERROR_CHECK(esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config));
ESP_ERROR_CHECK(esp_wifi_start());
xEventGroupWaitBits(wifi_event_group, CONNECTED_BIT, false, true, portMAX_DELAY);
ESP_LOGI(TAG, "Connected to AP");
}
static void websocket_event_handler(void *handler_args, esp_event_base_t base, int32_t event_id, void *event_data) {
switch (event_id) {
case WEBSOCKET_EVENT_CONNECTED:
ESP_LOGI(TAG, "websocket connected");
break;
case WEBSOCKET_EVENT_DISCONNECTED:
ESP_LOGI(TAG, "websocket disconnected");
break;
case WEBSOCKET_EVENT_DATA:
ESP_LOGI(TAG, "websocket received data");
break;
case WEBSOCKET_EVENT_ERROR:
ESP_LOGI(TAG, "websocket error");
break;
default:
break;
}
}
static void websocket_init(void) {
esp_websocket_client_config_t websocket_cfg = {
.uri = "wss://your_webrtc_server/ws",
.disable_auto_reconnect = true,
.keepalive_interval_ms = 5000,
.event_handle = websocket_event_handler,
};
ESP_ERROR_CHECK(esp_websocket_client_create(&websocket_cfg, &websocket));
ESP_ERROR_CHECK(esp_websocket_client_start(websocket));
}
static void http_event_handler(esp_http_client_event_t *evt) {
switch (evt->event_id) {
case HTTP_EVENT_ON_DATA:
ESP_LOGI(TAG, "http received data");
break;
case HTTP_EVENT_ON_FINISH:
ESP_LOGI(TAG, "http request finished");
break;
case HTTP_EVENT_ERROR:
ESP_LOGI(TAG, "http error");
break;
default:
break;
}
}
static void http_init(void) {
esp_http_client_config_t http_cfg = {
.url = "https://your_webrtc_server/start",
.event_handler = http_event_handler,
.method = HTTP_METHOD_POST,
};
client = esp_http_client_init(&http_cfg);
ESP_ERROR_CHECK(esp_http_client_perform(client));
}
void app_main() {
nvs_flash_init();
ESP_ERROR_CHECK(esp_camera_init(&config));
wifi_init_sta();
websocket_init();
http_init();
while (true) {
camera_fb_t *fb = esp_camera_fb_get();
if (!fb) {
ESP_LOGE(TAG, "failed to get camera frame buffer");
continue;
}
esp_err_t err = esp_websocket_client_send_binary(websocket, fb->buf, fb->len, portMAX_DELAY);
if (err != ESP_OK) {
ESP_LOGE(TAG, "failed to send data over websocket: %d", err);
}
esp_camera_fb_return(fb);
}
}
```
这个Demo需要用到ESP32的摄像头模块,推流的视频数据将从摄像头读取,并通过WebRTC协议发送到服务器。
你需要将代码中的`your_wifi_ssid`和`your_wifi_password`替换为你的WiFi名称和密码,将`your_webrtc_server`替换为你的WebRTC服务器地址。
请注意,这只是一个示例,实际情况下你需要根据你的需求进行修改和调整。
阅读全文
相关推荐

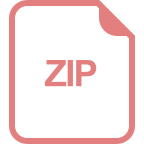
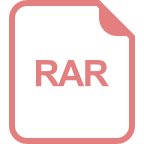
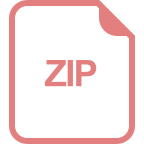
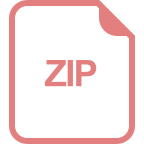
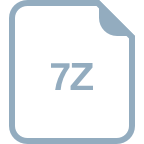
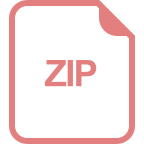
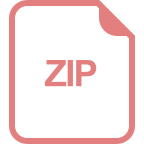
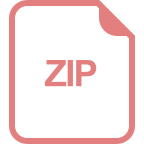
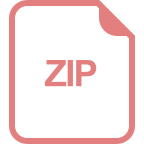
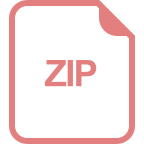
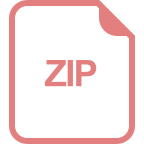
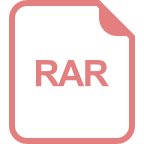
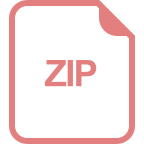