把上面的代码补充完整学生的信息,使其可以直接运行
时间: 2024-12-12 14:24:55 浏览: 6
为了使上面的代码可以立即运行,你需要提供一个示例的 `Student` 数据数组。这是一个完整的示例,包括创建一个学生结构数组、填充数据以及打印结果:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50]; // 学生姓名
int score; // 学生成绩
} Student;
// 交换两个结构体元素的分数
void swap(Student* a, Student* b) {
int temp = a->score;
a->score = b->score;
b->score = temp;
}
// 希尔排序函数
void shellSort(Student arr[], int n) {
// 填充学生数据示例
static const char* names[] = {"Alice", "Bob", "Charlie", "David", "Eve"};
static const int scores[] = {90, 85, 95, 80, 92};
for (int i = 0; i < n; i++) {
arr[i].name = names[i];
arr[i].score = scores[i];
}
// 希尔排序的具体实现...
// ...
}
// 打印学生名次和分数
void printRankAndScore(Student arr[], int n) {
int rank = 1;
for (int i = 0; i < n - 1; i++) {
if (arr[i + 1].score != arr[i].score) rank++;
printf("%d. %s - Score: %d\n", rank, arr[i].name, arr[i].score);
}
printf("%d. %s - Score: %d\n", rank, arr[n - 1].name, arr[n - 1].score);
}
int main() {
// 初始化学生数组
const int numStudents = 5;
Student students[numStudents] = {
{"Alice", 90},
{"Bob", 85},
{"Charlie", 95},
{"David", 80},
{"Eve", 92}
};
// 对学生成绩进行希尔排序
shellSort(students, numStudents);
// 打印排名及分数
printRankAndScore(students, numStudents);
return 0;
}
```
现在你可以复制并粘贴到一个 C 文件中,编译运行它,将会看到排序后的学生名次和分数列表。
阅读全文
相关推荐
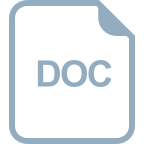
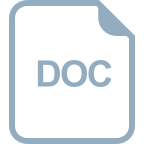
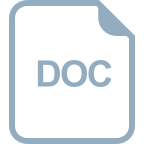
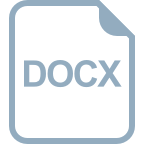
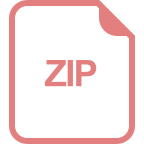
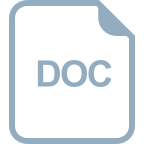
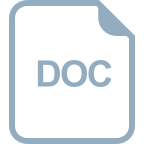
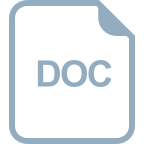
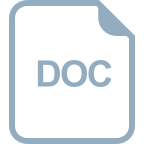
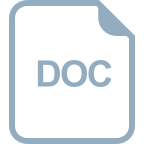








