写一个ble 获取体温数据的蓝牙app代码
时间: 2024-03-02 14:48:53 浏览: 74
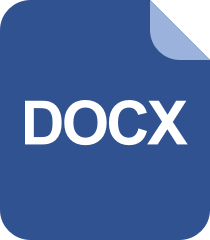
BeneCheck使用蓝牙模块BLE获取血糖尿酸胆固醇数据-

这里给出一个简单的 Android BLE 获取体温数据的蓝牙app代码示例。
首先,需要在项目的 `AndroidManifest.xml` 文件中添加 BLE 权限:
```xml
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
```
然后,在 `MainActivity.java` 中,进行 BLE 的初始化、连接和数据读取:
```java
public class MainActivity extends AppCompatActivity {
private BluetoothManager bluetoothManager;
private BluetoothAdapter bluetoothAdapter;
private BluetoothGatt bluetoothGatt;
private BluetoothGattCharacteristic temperatureCharacteristic;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
bluetoothAdapter = bluetoothManager.getAdapter();
BluetoothDevice device = bluetoothAdapter.getRemoteDevice("00:11:22:33:44:55");
bluetoothGatt = device.connectGatt(this, false, gattCallback);
}
private BluetoothGattCallback gattCallback = new BluetoothGattCallback() {
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 断开连接
}
}
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
BluetoothGattService service = gatt.getService(UUID.fromString("00001809-0000-1000-8000-00805f9b34fb"));
temperatureCharacteristic = service.getCharacteristic(UUID.fromString("00002A1C-0000-1000-8000-00805f9b34fb"));
gatt.setCharacteristicNotification(temperatureCharacteristic, true);
}
}
@Override
public void onCharacteristicChanged(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic) {
if (characteristic == temperatureCharacteristic) {
// 解析温度数据
byte[] data = characteristic.getValue();
float temperature = ByteBuffer.wrap(data).order(ByteOrder.LITTLE_ENDIAN).getFloat();
Log.d(TAG, "Temperature: " + temperature);
}
}
};
}
```
在上面的代码中,我们首先通过 `BluetoothManager` 和 `BluetoothAdapter` 初始化 BLE,连接指定的设备,然后在 GATT 回调函数中,发现可用服务和特征,设置特征的通知,最后在特征值改变时读取并解析温度数据。
需要注意的是,这只是一个简单的示例,实际的实现可能会更加复杂,需要根据具体的硬件设计和数据格式进行适当的修改。
阅读全文
相关推荐
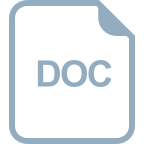
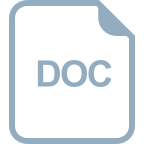
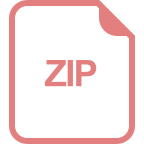
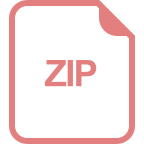
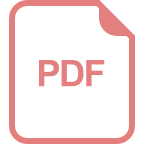
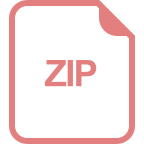
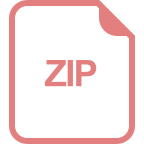
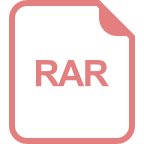
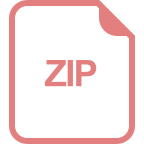
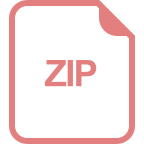
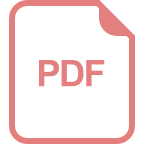
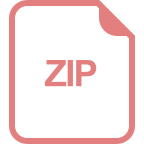
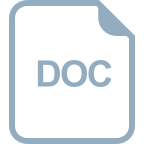
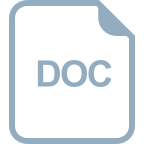
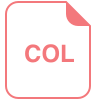
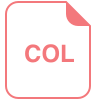
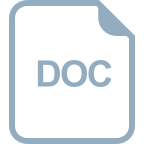
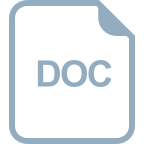