51单片机接收计算机通过串口传输过来的字符,同时做如下操作: 1 将接收到的字符回传给计算机,其中的小写字母转换为大些字母,其他回传字符不变。 2 将接收到的可显示字符显示在LCD1602液晶屏上。
时间: 2024-05-13 22:13:27 浏览: 11
以下是基于STC89C52单片机的代码:
```c
#include <reg52.h>
#include <stdio.h>
#include <stdlib.h>
// 宏定义
#define LCD1602_DB P0
#define LCD1602_RS P2_0
#define LCD1602_RW P2_1
#define LCD1602_EN P2_2
// 函数声明
void init_serial();
void init_lcd();
void write_command(unsigned char command);
void write_data(unsigned char data);
void write_string(unsigned char *string);
void delay(unsigned int ms);
// 主函数
void main() {
unsigned char ch;
init_serial();
init_lcd();
write_string("Ready to receive...");
while (1) {
if (RI) {
ch = SBUF;
RI = 0;
// 将接收到的字符回传给计算机
if (ch >= 'a' && ch <= 'z') {
ch -= 32;
}
SBUF = ch;
while (!TI);
TI = 0;
// 将接收到的可显示字符显示在LCD1602液晶屏上
if (ch >= 32 && ch <= 126) {
write_data(ch);
}
}
}
}
// 初始化串口
void init_serial() {
TMOD = 0x20; // 定时器1工作在模式2
TH1 = 0xfd; // 波特率为9600
TL1 = 0xfd;
TR1 = 1; // 启动定时器1
SM0 = 0; // 串口工作在模式1
SM1 = 1;
REN = 1; // 启动接收
EA = 1; // 开启总中断
ES = 1; // 开启串口中断
}
// 初始化LCD1602液晶屏
void init_lcd() {
delay(15);
write_command(0x38); // 设置16x2显示,5x7点阵,8位数据总线
delay(5);
write_command(0x38);
delay(5);
write_command(0x38);
delay(5);
write_command(0x08); // 关闭显示
delay(5);
write_command(0x01); // 清屏
delay(5);
write_command(0x06); // 设置文字不移动,光标右移
delay(5);
write_command(0x0c); // 打开显示,关闭光标
delay(5);
}
// 写入命令
void write_command(unsigned char command) {
LCD1602_RS = 0;
LCD1602_RW = 0;
LCD1602_DB = command;
LCD1602_EN = 1;
delay(1);
LCD1602_EN = 0;
}
// 写入数据
void write_data(unsigned char data) {
LCD1602_RS = 1;
LCD1602_RW = 0;
LCD1602_DB = data;
LCD1602_EN = 1;
delay(1);
LCD1602_EN = 0;
}
// 写入字符串
void write_string(unsigned char *string) {
while (*string != '\0') {
write_data(*string++);
}
}
// 延时函数
void delay(unsigned int ms) {
unsigned int i, j;
for (i = 0; i < ms; i++) {
for (j = 0; j < 114; j++);
}
}
// 串口中断服务函数
void serial_isr() interrupt 4 {
if (RI) {
RI = 0;
}
if (TI) {
TI = 0;
}
}
```
相关推荐
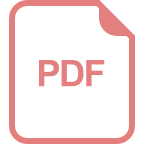
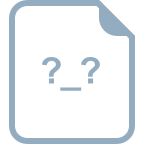














