vb.net 解析视频文件的元数据 gps
时间: 2023-09-13 17:08:31 浏览: 140
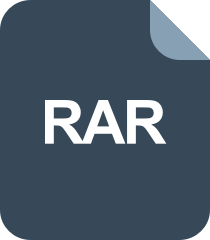
vb.NET gps数据读取
在 VB.NET 中解析视频元数据和 GPS 数据,可以使用一些第三方库,比如 FFmpeg 和 Exiftool。以下是使用 FFmpeg 和 Exiftool 的示例代码:
1. 解析视频元数据
使用 FFmpeg 库可以解析视频文件的元数据,可以使用 NuGet 来安装 FFmpeg 库。以下是示例代码:
```vb.net
Imports FFmpeg.AutoGen
' 初始化 FFmpeg 库
FFmpegBinariesHelper.RegisterFFmpegBinaries()
' 打开视频文件
Dim inputFile As String = "path/to/video.mp4"
Dim inputFormatContext As AVFormatContext = Nothing
FFmpegInvoke.avformat_open_input(inputFormatContext, inputFile, Nothing, Nothing)
' 读取视频流信息
FFmpegInvoke.avformat_find_stream_info(inputFormatContext, Nothing)
' 获取视频元数据
Dim metadata As Dictionary(Of String, String) = New Dictionary(Of String, String)()
For i As Integer = 0 To inputFormatContext.nb_streams - 1
Dim streamMetadata As AVDictionary = inputFormatContext.streams(i).metadata
While streamMetadata IsNot Nothing
Dim key As String = FFmpegInvoke.av_dict_get_key(streamMetadata)
Dim value As String = FFmpegInvoke.av_dict_get(streamMetadata, key, Nothing, 0)
metadata.Add(key, value)
streamMetadata = streamMetadata.Next
End While
Next
' 关闭视频文件
FFmpegInvoke.avformat_close_input(inputFormatContext)
' 输出视频元数据
For Each pair As KeyValuePair(Of String, String) In metadata
Console.WriteLine("{0}: {1}", pair.Key, pair.Value)
Next
```
2. 解析 GPS 数据
使用 Exiftool 库可以解析视频文件中的 GPS 数据,可以使用 NuGet 来安装 Exiftool 库。以下是示例代码:
```vb.net
Imports PhilHarvey.ExifToolLib
' 打开视频文件
Dim inputFile As String = "path/to/video.mp4"
Dim exiftool As ExifTool = New ExifTool()
Dim tags As String = exiftool.GetTags(inputFile)
' 解析 GPS 数据
Dim gps As String = ""
If tags.Contains("GPS Latitude") AndAlso tags.Contains("GPS Longitude") Then
Dim latitude As Double = GetGpsCoordinate(tags, "GPS Latitude")
Dim longitude As Double = GetGpsCoordinate(tags, "GPS Longitude")
Dim latitudeRef As String = GetGpsRef(tags, "GPS Latitude Ref")
Dim longitudeRef As String = GetGpsRef(tags, "GPS Longitude Ref")
gps = String.Format("{0} {1}, {2} {3}", latitude, latitudeRef, longitude, longitudeRef)
End If
' 关闭视频文件
exiftool.Dispose()
' 输出 GPS 数据
Console.WriteLine("GPS: {0}", gps)
' 获取 GPS 坐标
Private Function GetGpsCoordinate(tags As String, tagName As String) As Double
Dim coordinate As Double = 0
Dim parts As String() = tags.Split(New String() {tagName & " : "}, StringSplitOptions.None)
If parts.Length > 1 Then
Dim coordinateParts As String() = parts(1).Split(New Char() {","c, " "c}, StringSplitOptions.RemoveEmptyEntries)
If coordinateParts.Length = 3 Then
Dim degrees As Double = Double.Parse(coordinateParts(0))
Dim minutes As Double = Double.Parse(coordinateParts(1))
Dim seconds As Double = Double.Parse(coordinateParts(2))
coordinate = degrees + (minutes / 60) + (seconds / 3600)
End If
End If
Return coordinate
End Function
' 获取 GPS 参考方向
Private Function GetGpsRef(tags As String, tagName As String) As String
Dim ref As String = ""
Dim parts As String() = tags.Split(New String() {tagName & " : "}, StringSplitOptions.None)
If parts.Length > 1 Then
ref = parts(1).Trim()
End If
Return ref
End Function
```
阅读全文
相关推荐
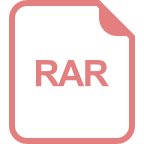
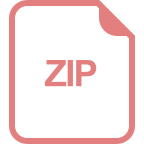
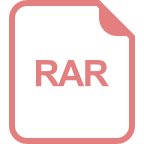
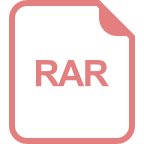
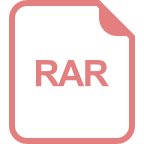
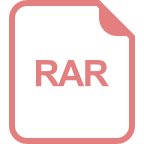
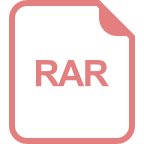
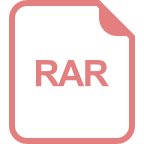
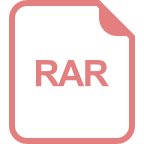
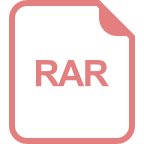
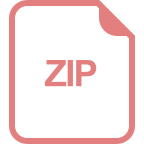
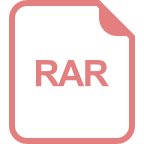
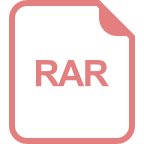
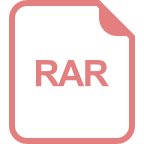
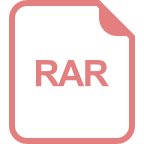