#include<stdio.h> #include<string.h> union score { int score1; char score2[10]; }; struct data { char name[10]; char cls[50]; char type; union score a; }; int main() { int n,i; struct data person[20]; char str[10]; scanf("%d",&n); for(i=0;i<n;i++) { scanf("%s%s%c",&person[i].name,&person[i].cls,&person[i].type); if(person[i].type=='0') scanf("%d",&person[i].a.score1); else scanf("%s",&person[i].a.score2); } // printf("%s %s %c %d",person[0].name,person[0].cls,person[0].type,person[0].a.score1); printf("%d",person[0].a.score1); /* strcpy(str,person[n-1].name); for(i=0;i<n;i++) { if(strcmp(person[i].name,str)==0) { printf("%s %s %c",person[i].name,person[i].cls,person[i].type); if(person[i].type=='0') printf("%d",person[i].a.score1); else printf("%s",person[i].a.score2); } printf("%c",'\n'); } */ return 0; }
时间: 2023-12-06 22:41:29 浏览: 66
这段代码定义了一个结构体和联合体,用于存储学生的姓名、班级、类型和成绩。其中成绩可以是整型或者字符串类型。接着在主函数中,通过输入学生数量和每个学生的信息,来赋值结构体和联合体。最后输出第一个学生的整型成绩。
需要注意的是,代码中有一些问题。比如在输入姓名、班级和类型时,需要加上一个空格的占位符,否则会出现错误;另外,注释掉的部分代码可能会导致输出多余的换行符,需要注意。
相关问题
有若干个学生的成绩数据,其中成绩有百分制与五分制(优、良、中、及格和不及格)。学生的数据中包括:学号、课程名、成绩类型、成绩。要求用同一个表格来处理,设计百分制与五级分制通用的表格 步骤: 1) 定义共用体类型; 2) 定义学生结构体类型,其中成绩是共用体类型; 3) 运行程序时随机输入测试数据,成绩类型1代表百分制,5代表五分制: 101 C语言 1 80 101 体育 5 良 102 C语言 1 75 102 体育 5 优 3)输出学生的学号、课程名和成绩信息。
代码如下:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义共用体类型
typedef union {
int score_percent; // 百分制成绩
char score_five[3]; // 五分制成绩:优、良、中、及格、不及格
} Score;
// 定义学生结构体类型
typedef struct {
int stu_id; // 学号
char course_name[20]; // 课程名
int score_type; // 成绩类型:1代表百分制,5代表五分制
Score score; // 成绩
} Student;
int main() {
Student stu[3]; // 存储3个学生的数据
int i;
// 随机输入测试数据
for (i = 0; i < 3; i++) {
printf("请输入第%d个学生的数据:\n", i+1);
printf("学号:");
scanf("%d", &stu[i].stu_id);
printf("课程名:");
scanf("%s", stu[i].course_name);
printf("成绩类型(1代表百分制,5代表五分制):");
scanf("%d", &stu[i].score_type);
if (stu[i].score_type == 1) {
printf("百分制成绩:");
scanf("%d", &stu[i].score.score_percent);
} else if (stu[i].score_type == 5) {
printf("五分制成绩(优、良、中、及格、不及格):");
scanf("%s", stu[i].score.score_five);
} else {
printf("错误的成绩类型!\n");
exit(1);
}
}
// 输出学生的学号、课程名和成绩信息
printf("\n学号\t课程名\t成绩\n");
for (i = 0; i < 3; i++) {
printf("%d\t%s\t", stu[i].stu_id, stu[i].course_name);
if (stu[i].score_type == 1) {
printf("%d\n", stu[i].score.score_percent);
} else if (stu[i].score_type == 5) {
printf("%s\n", stu[i].score.score_five);
}
}
return 0;
}
c语言使用结构体和共用体编写程序,输入n个学生的学号和成绩(有1位小数),按成绩从高到低排序输出,n小于50,学号小于10位。
好的,这是一个关于 C 语言编程的问题。我可以回答这个问题。下面是代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define N 50
#define MAX_ID_LEN 10
struct Student {
char id[MAX_ID_LEN + 1];
float score;
};
union Data {
struct Student student;
char buffer[sizeof(struct Student)];
};
int cmp(const void *a, const void *b) {
struct Student *s1 = (struct Student *)a;
struct Student *s2 = (struct Student *)b;
if (s1->score > s2->score) {
return -1;
} else if (s1->score < s2->score) {
return 1;
} else {
return strcmp(s1->id, s2->id);
}
}
int main() {
int n;
union Data data[N];
scanf("%d", &n);
for (int i = 0; i < n; i++) {
scanf("%s %f", data[i].student.id, &data[i].student.score);
}
qsort(data, n, sizeof(union Data), cmp);
for (int i = 0; i < n; i++) {
printf("%s %.1f\n", data[i].student.id, data[i].student.score);
}
return 0;
}
```
这个程序使用结构体和共用体来存储学生的信息,然后使用快速排序算法按成绩从高到低排序,最后输出成绩排名。
阅读全文
相关推荐
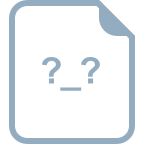
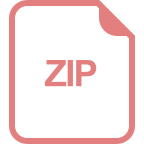
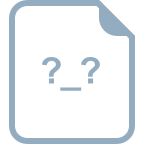




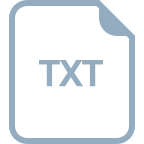
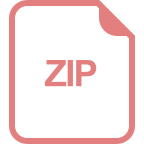
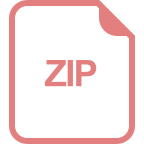
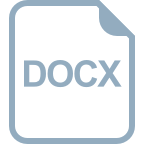
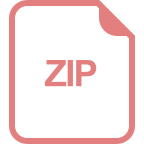
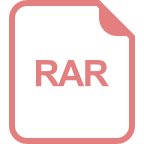
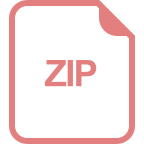
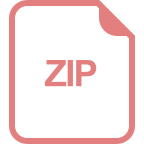
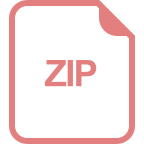