设计一个C语言函数 获取Linux开机的时间
时间: 2023-11-25 11:05:45 浏览: 96
您可以使用C语言中time函数来获取Linux系统开机的时间。time函数返回自1970年1月1日以来经过的秒数,可以通过将time函数返回值作为参数传递给localtime函数来获取本地时间,最后再通过strftime函数将时间格式化成字符串。
下面是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
time_t raw_time;
struct tm *time_info;
char time_str[100];
time(&raw_time);
time_info = localtime(&raw_time);
strftime(time_str, sizeof(time_str), "%Y-%m-%d %H:%M:%S", time_info);
printf("Linux系统开机时间为:%s\n", time_str);
return 0;
}
```
该程序将当前系统时间格式化成"XXXX-XX-XX XX:XX:XX"的字符串输出,即为Linux系统开机的时间。
相关问题
设计一个C语言函数 获取Linux开机时的时间
可以使用time函数来获取当前时间,而Linux开机的时间则可以通过读取/proc/uptime文件获取。因此,设计一个C语言函数获取Linux开机时的时间的方法如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double get_boot_time() {
char buf[128];
double boot_time;
FILE *fp = fopen("/proc/uptime", "r");
if (fp == NULL) {
perror("Error opening /proc/uptime");
exit(EXIT_FAILURE);
}
if (fgets(buf, sizeof(buf), fp) == NULL) {
perror("Error reading /proc/uptime");
exit(EXIT_FAILURE);
}
fclose(fp);
boot_time = strtod(buf, NULL);
return boot_time;
}
```
这个函数会返回Linux系统开机以来的时间,单位是秒。
设计一个C语言函数 获取Linux开机的时刻
可以使用C语言中的time函数和Linux系统中的uptime命令来获取Linux开机的时刻。以下是一个获取Linux开机时刻的示例C语言函数:
```c
#include <stdio.h>
#include <stdlib.h>
void get_boot_time(char *boot_time) {
FILE *fp;
char uptime[20];
// 执行uptime命令并读取输出
fp = popen("uptime -s", "r");
if (fp == NULL) {
printf("Error: failed to execute uptime command.\n");
exit(1);
}
fgets(uptime, sizeof(uptime), fp);
pclose(fp);
// 获取开机时刻并保存到boot_time中
snprintf(boot_time, 20, "%s", uptime);
}
int main() {
char boot_time[20];
// 获取开机时刻
get_boot_time(boot_time);
printf("Linux boot time: %s", boot_time);
return 0;
}
```
该函数使用popen函数执行uptime命令并读取输出,然后使用snprintf函数从输出中提取出开机时刻并保存到boot_time中。最后在主函数中输出boot_time即可。
阅读全文
相关推荐
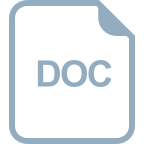
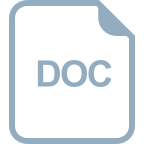
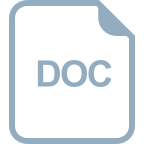
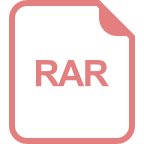
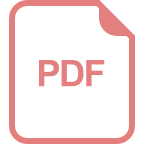
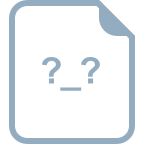
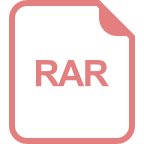
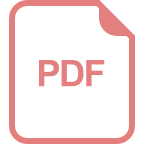
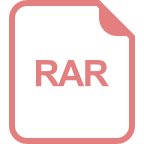
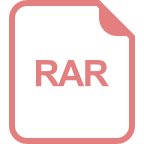
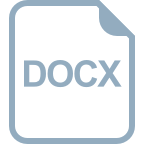
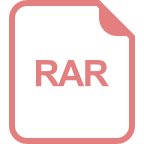
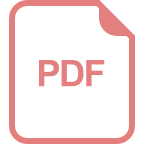
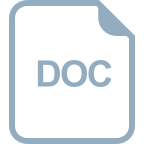


