6-2 2020Student类与对象数组分数 10 全屏浏览题目 切换布局 作者 shou 单位 上海海洋大学 本题要求定义一个简单的学生类,数据成员有学号、姓名和一门课成绩分数,函数成员的原型见给出的代码,请给出函数成员的类外完整实现。 此外,还需要实现一个显示学生对象数组的函数。函数接口定义如下: void disp_students(Student a[], int n); 其中,a 为学生对象数组,n为对象数组元素个数。
时间: 2024-02-03 19:11:56 浏览: 41
以下是完整的Student类与函数实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
string id; // 学号
string name; // 姓名
int score; // 一门课成绩分数
public:
Student(string id, string name, int score) {
this->id = id;
this->name = name;
this->score = score;
}
void setId(string id) {
this->id = id;
}
void setName(string name) {
this->name = name;
}
void setScore(int score) {
this->score = score;
}
string getId() {
return id;
}
string getName() {
return name;
}
int getScore() {
return score;
}
};
void disp_students(Student a[], int n) {
for (int i = 0; i < n; i++) {
cout << "学号:" << a[i].getId() << ",姓名:" << a[i].getName() << ",成绩:" << a[i].getScore() << endl;
}
}
int main() {
Student s1("1001", "张三", 90);
Student s2("1002", "李四", 80);
Student s3("1003", "王五", 70);
Student s4("1004", "赵六", 60);
Student students[] = {s1, s2, s3, s4};
disp_students(students, 4);
return 0;
}
```
以上代码定义了一个Student类,其中包括学号、姓名和成绩三个数据成员,以及对应的set和get函数。在主函数中创建了四个学生对象,并将它们存入一个数组中,最后调用disp_students函数输出这些学生的信息。
阅读全文
相关推荐
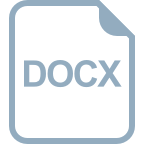
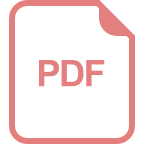
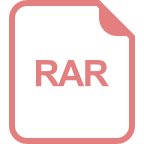














